c++创建两个二维整数矩阵, 要求输出矩阵, 并计算两个矩阵的加法、乘法结果及其计算时间。尽量简便
时间: 2024-09-22 12:05:46 浏览: 39
在C++中,你可以使用`std::vector`来创建二维整数矩阵,并利用`chrono`库来测量时间和进行矩阵运算。下面是一个简单的示例,展示了如何创建矩阵、输出矩阵、以及实现矩阵的加法和乘法:
```cpp
#include <iostream>
#include <vector>
#include <chrono>
// 定义矩阵类
class Matrix {
private:
std::vector<std::vector<int>> data;
int rows, cols;
public:
// 构造函数
Matrix(int r, int c) : rows(r), cols(c) { data.resize(rows, std::vector<int>(cols, 0)); }
// 输出矩阵
void display() const {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j)
std::cout << data[i][j] << " ";
std::cout << "\n";
}
}
// 加法
Matrix operator+(const Matrix& other) {
if (rows != other.rows || cols != other.cols) throw std::runtime_error("Matrices must have the same dimensions for addition");
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j)
result.data[i][j] = data[i][j] + other.data[i][j];
}
return result;
}
// 乘法(假设是元素级乘法)
Matrix operator*(const Matrix& other) {
if (cols != other.rows) throw std::runtime_error("Incompatible matrix dimensions for multiplication");
Matrix result(rows, other.cols);
auto start = std::chrono::high_resolution_clock::now();
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < other.cols; ++j) {
int sum = 0;
for (int k = 0; k < cols; ++k)
sum += data[i][k] * other.data[k][j];
result.data[i][j] = sum;
}
}
auto end = std::chrono::high_resolution_clock::now();
double time_spent = std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() / 1e6; // 转换为毫秒
std::cout << "Time taken for multiplication: " << time_spent << " ms\n";
return result;
}
};
int main() {
int r, c;
std::cout << "Enter number of rows and columns for each matrix: ";
std::cin >> r >> c;
Matrix m1(r, c), m2(r, c);
// 获取用户输入并填充矩阵
std::cout << "Enter elements for matrix 1:\n";
for (auto &row : m1.data)
for (auto &element : row)
std::cin >> element;
std::cout << "Enter elements for matrix 2:\n";
for (auto &row : m2.data)
for (auto &element : row)
std::cin >> element;
// 输出和运算
std::cout << "Matrix 1:\n" << m1 << "\n";
std::cout << "Matrix 2:\n" << m2 << "\n";
m1.display(); // 输出第一个矩阵
m2.display(); // 输出第二个矩阵
Matrix sum = m1 + m2;
std::cout << "Sum:\n" << sum << "\n";
Matrix product = m1 * m2;
std::cout << "Product:\n" << product << "\n";
return 0;
}
```
阅读全文
相关推荐
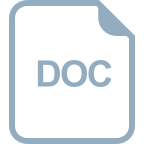
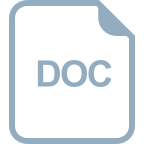
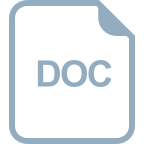
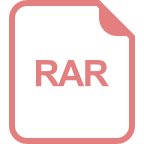
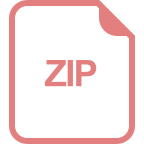
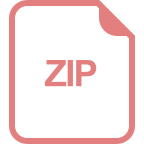
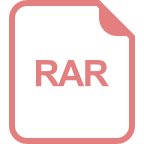
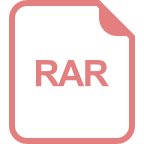
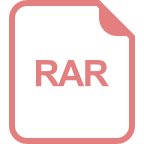
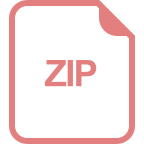
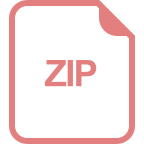
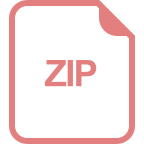
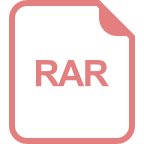
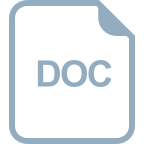
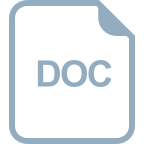
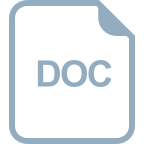
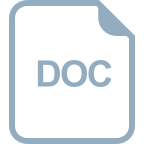
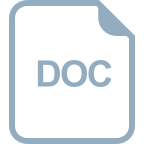
