如何用C语言编写一个实现老鼠走迷宫的程序?
时间: 2024-12-18 10:31:56 浏览: 9
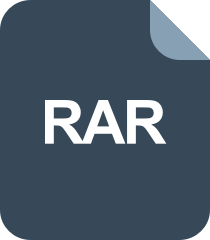
走迷宫C语言
在C语言中编写一个老鼠走迷宫的程序通常涉及到搜索算法,比如广度优先搜索(Breadth First Search, BFS)或深度优先搜索(Deep First Search, DFS),以及动态规划或者回溯法。这里我会简单描述一个基于BFS的基本步骤:
1. **创建迷宫矩阵**:用二维数组表示迷宫,0表示可以通行,1表示墙壁。
2. **初始化老鼠位置**:设老鼠起始点(例如左上角)为`(0, 0)`。
3. **设置目标点**:如终点为`(M-1, N-1)`,其中`M`和`N`分别是迷宫的行数和列数。
4. **BFS遍历**:
- 创建一个队列,将起始点加入。
- 当队列不为空时:
- 弹出队首节点作为当前节点。
- 检查这个节点是否为目标节点。如果是,则找到出路,结束搜索。
- 否则,检查其上下左右四个相邻节点:
- 如果它们是空的(即未访问过),标记为已访问并加入队列。
5. **路径记录**:在遍历时,如果找到一个新节点,将其前一个节点(即移动方向)加入到“路径”数组中,以便后续回溯路径。
6. **输出结果**:当找到出口时,逆序打印路径,展示老鼠走出迷宫的路线。
```c
#include <stdio.h>
#include <stdlib.h>
#define ROW 8 // 迷宫大小
#define COL 8
// 省略迷宫矩阵定义...
void bfs(int maze[][COL], int start_row, int start_col, int end_row, int end_col) {
int visited[ROW][COL] = {0};
queue_t *q = create_queue();
enqueue(q, start_row, start_col);
visited[start_row][start_col] = 1;
while (!is_empty(q)) {
int cur_row, cur_col;
dequeue(q, &cur_row, &cur_col);
if (cur_row == end_row && cur_col == end_col) {
print_path(visited, start_row, start_col, end_row, end_col);
return;
}
for (int direction = 0; direction < 4; ++direction) {
int next_row = cur_row + move_directions[direction][0];
int next_col = cur_col + move_directions[direction][1];
if (next_row >= 0 && next_row < ROW && next_col >= 0 && next_col < COL &&
!maze[next_row][next_col] && !visited[next_row][next_col]) {
visited[next_row][next_col] = 1;
enqueue(q, next_row, next_col);
}
}
}
}
// ... 其他函数省略 ...
int main() {
int maze[ROW][COL]; // 初始化迷宫
bfs(maze, 0, 0, ROW - 1, COL - 1); // 调用搜索函数
return 0;
}
```
阅读全文
相关推荐
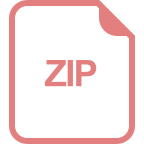
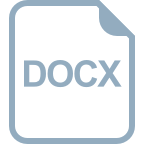

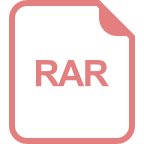
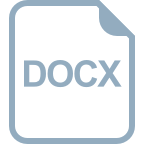
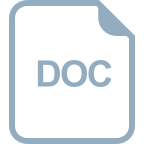




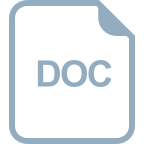
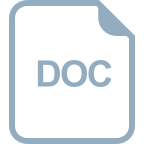
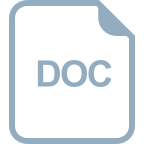
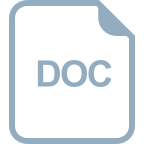
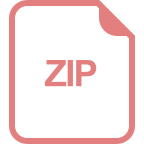