如何为具有不规则边界的自定义Widget设置背景图片,以确保在显示时无黑色边框?
时间: 2024-12-25 18:23:55 浏览: 4
在Qt中,为自定义QWidget设置不规则边界背景图片并避免黑色边框,可以采用以下方法:
1. **使用QPainter和QRegion**:
- 继承自QWidget,并在paintEvent()事件处理程序中画出背景。
- 初始化一个QPainter对象,选择图片作为前景色:
```cpp
QPainter painter(this);
QPixmap pixmap("your_image.png");
painter.setBrush(QColor(pixmap.color(0, 0)));
```
- 获取窗口的不规则形状(比如通过setMask()设置),然后填充:
```cpp
QRegion region = this->mask();
painter.fillRect(region.boundingRect(), pixmap);
```
2. **使用QImage和QPainter**:
- 创建一个QImage对象,以原始图片大小创建并从图片提取颜色数据。
- 调整图像的颜色以便匹配窗口边框,如将边框颜色设为透明:
```cpp
QImage image = pixmap.convertToFormat(QImage::Format_ARGB32_Premultiplied);
QColor borderColor = window()->palette().color(QPalette::Base).darker(); // 黑色变暗一点,避免直接透明
image.fill(borderColor.rgb());
```
- 将QImage转换回QPixmap,并设置到窗口上:
```cpp
QPixmap pixmapFromImage(image);
QWidget::setMask(QRegion(this->geometry()));
setAutoFillBackground(true);
setStyleSheet("background-image: url(:/" + pixmapFromImage.save("custom_background.png") + ");");
```
3. **注意边缘抗锯齿(Anti-Aliasing)**:
- 为了减少锯齿边缘,确保QPainter和QImage的抗锯齿功能启用(对于QImage,可以使用QImage::alphaChannel())。
在上述方法中,确保将"your_image.png"替换为你实际的图片文件名,并根据需求调整边框颜色。使用setMask()和auto-fill background可以帮助隐藏默认的白色边框。
阅读全文
相关推荐
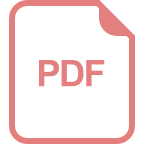
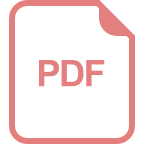
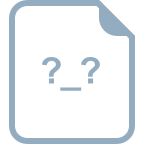















