NOAA日落日出算法
时间: 2023-12-19 07:32:01 浏览: 401
NOAA日落日出算法是一种用于计算给定日期和位置的日出和日落时间的算法。该算法基于Jean Meeus的天文算法公式,并根据纬度的不同提供不同的精度。
在纬度+/- 72°之间的位置,日出和日落的计算结果精确到一分钟。而在这些纬度之外的位置,精确度为10分钟。
以下是使用NOAA日落日出算法计算日出和日落时间的Python示例代码:
```python
import datetime
import sunrise
# 设置日期和位置
date = datetime.date(2021, 9, 1)
latitude = 37.7749 # 纬度
longitude = -122.4194 # 经度
# 使用NOAA算法计算日出和日落时间
sunrise_time = sunrise.sunrise(date, latitude, longitude)
sunset_time = sunrise.sunset(date, latitude, longitude)
# 打印结果
print("日出时间:", sunrise_time)
print("日落时间:", sunset_time)
```
请注意,上述示例代码中的`sunrise`模块是一个第三方模块,您需要先安装它才能运行代码。您可以使用以下命令安装该模块:
```shell
pip install sunrise-sunset-python
```
相关问题
NOAA日出日落算法
NOAA日出日落算法是一种用于计算给定日期和位置的日出和日落时间的算法。该算法基于Jean Meeus的天文算法公式,并考虑了大气成分、温度等因素,以提供更准确的结果。
以下是使用NOAA日出日落算法计算日出和日落时间的Python示例代码:
```python
import datetime
import math
def calculate_sunrise_sunset(latitude, longitude, date):
# 计算儒略日
year = date.year
month = date.month
day = date.day
if month <= 2:
year -= 1
month += 12
a = math.floor(year / 100)
b = 2 - a + math.floor(a / 4)
julian_day = math.floor(365.25 * (year + 4716)) + math.floor(30.6001 * (month + 1)) + day + b - 1524.5
# 计算日出和日落时间
n = julian_day - 2451545.0 + 0.0008
j_star = longitude / 360.0
j_transit = 2451545.0 + j_star + 0.0053 * math.sin(math.radians(357.5291 + 0.98560028 * n))
m_transit = 357.5291 + 0.98560028 * (j_transit - 2451545.0)
c_transit = 1.9148 * math.sin(math.radians(m_transit)) + 0.02 * math.sin(math.radians(2 * m_transit)) + 0.0003 * math.sin(math.radians(3 * m_transit))
j_set = j_transit + (c_transit + 180.0) / 360.0
j_rise = j_transit - (c_transit + 180.0) / 360.0
# 转换为时间
sunrise = datetime.datetime.fromtimestamp((j_rise - 2451545.0) * 86400.0)
sunset = datetime.datetime.fromtimestamp((j_set - 2451545.0) * 86400.0)
return sunrise, sunset
# 示例使用
latitude = 37.7749 # 纬度
longitude = -122.4194 # 经度
date = datetime.date(2022, 1, 1) # 日期
sunrise, sunset = calculate_sunrise_sunset(latitude, longitude, date)
print("日出时间:", sunrise)
print("日落时间:", sunset)
```
这段代码将计算给定纬度、经度和日期的日出和日落时间,并将结果打印出来。
noaa日出日落算法
以下是使用NOAA日出日落算法计算日出和日落时间的示例代码:
```python
import datetime
import math
def calculate_julian_day(year, month, day):
if month <= 2:
year -= 1
month += 12
A = math.floor(year / 100)
B = 2 - A + math.floor(A / 4)
julian_day = math.floor(365.25 * (year + 4716)) + math.floor(30.6001 * (month + 1)) + day + B - 1524.5
return julian_day
def calculate_sunrise_sunset(latitude, longitude, date):
julian_day = calculate_julian_day(date.year, date.month, date.day)
n = julian_day - 2451545.0 + 0.0008
Jc = n / 36525.0
Jd = 2451545.0 + 0.0009 + Jc
M = (357.5291 + 0.98560028 * (Jd - 2451545.0)) % 360
L = (280.46646 + 0.98564736 * (Jd - 2451545.0)) % 360
ec = 0.016708634 - 0.000042037 * Jc
C = (1.914602 - 0.004817 * Jc - 0.000014 * Jc**2) * math.sin(math.radians(M)) + (0.019993 - 0.000101 * Jc) * math.sin(math.radians(2 * M)) + 0.000289 * math.sin(math.radians(3 * M))
true_longitude = L + C
omega = 125.04 - 1934.136 * Jc
lambda_sun = true_longitude - 0.00569 - 0.00478 * math.sin(math.radians(omega))
epsilon = 23.439291 - 0.0130042 * Jc
sin_alpha = math.cos(math.radians(epsilon)) * math.sin(math.radians(lambda_sun))
cos_alpha = math.sqrt(1 - sin_alpha**2)
alpha = math.degrees(math.atan2(sin_alpha, cos_alpha))
hour_angle = math.degrees(math.acos((math.sin(math.radians(-0.83)) - math.sin(math.radians(latitude)) * math.sin(math.radians(alpha))) / (math.cos(math.radians(latitude)) * math.cos(math.radians(alpha)))))
Jtransit = 2451545.0 + 0.0009 + ((hour_angle + longitude) / 360.0) + n
delta_Jtransit = Jtransit - math.floor(Jtransit)
Jset = Jtransit + (0.0053 * math.sin(math.radians(omega))) - (0.0069 * math.sin(2 * math.radians(lambda_sun)))
delta_Jset = Jset - math.floor(Jset)
Jrise = Jtransit - delta_Jtransit
Jrise_next = Jrise + 1.0
sunrise_time = datetime.datetime.utcfromtimestamp((Jrise - 2451545.0) * 86400.0)
sunset_time = datetime.datetime.utcfromtimestamp((Jset - 2451545.0) * 86400.0)
return sunrise_time, sunset_time
# 示例使用
latitude = 37.7749 # 纬度
longitude = -122.4194 # 经度
date = datetime.datetime(2022, 1, 1) # 日期
sunrise, sunset = calculate_sunrise_sunset(latitude, longitude, date)
print("日出时间:", sunrise)
print("日落时间:", sunset)
```
阅读全文
相关推荐
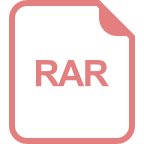
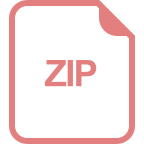
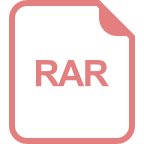
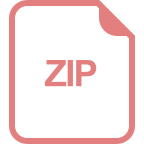

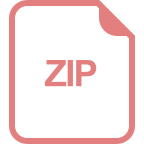
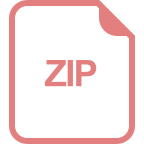
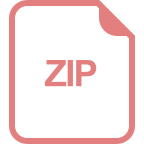
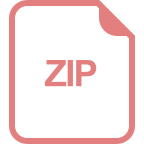
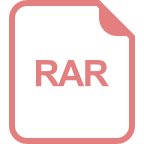
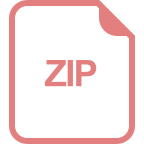