python 判断list为空的函数
时间: 2023-12-30 20:05:05 浏览: 31
Python中可以使用以下函数来判断一个列表是否为空:
```python
def is_list_empty(lst):
if len(lst) == 0:
return True
else:
return False
```
以上代码定义了一个名为 `is_list_empty` 的函数,它接受一个列表作为参数 `lst`。函数内部使用 `len()` 函数来获取列表的长度,如果长度为0,则说明列表为空,函数返回 `True`,否则返回 `False`。
你可以调用这个函数并传入一个列表来判断它是否为空。例如:
```python
my_list = []
if is_list_empty(my_list):
print("List is empty")
else:
print("List is not empty")
```
以上代码中,我们定义了一个空列表 `my_list`,然后调用 `is_list_empty()` 函数来判断该列表是否为空,并根据结果输出相应的信息。如果运行以上代码,将会输出 "List is empty",表示列表为空。
相关问题
python 判断list是否为空
以下是两种判断列表是否为空的方法:
1. 使用len()函数判断列表是否为空[^1]:
```python
list = []
if len(list) == 0:
print('list is empty')
```
2. 使用if语句判断列表是否为空[^2]:
```python
list_temp = []
if not list_temp:
print('list_temp is empty')
```
python 判断不为空
在Python中,可以使用以下方法来判断对象是否不为空:
1. 使用if语句判断对象是否不为空,例如:
```
# 判断列表是否不为空
my_list = [1, 2, 3]
if my_list:
print("列表不为空")
# 判断字符串是否不为空
my_str = "hello"
if my_str:
print("字符串不为空")
```
2. 使用bool()函数判断对象是否不为空,例如:
```
# 判断列表是否不为空
my_list = [1, 2, 3]
if bool(my_list) == True:
print("列表不为空")
# 判断字符串是否不为空
my_str = "hello"
if bool(my_str) == True:
print("字符串不为空")
```
注意,以上两种方法在判断非空对象时是等价的,都可以判断对象是否不为空。同时,对于列表、字符串、字典等集合类型的对象,如果其中至少有一个元素不为空,则也可以认为该对象不为空。
相关推荐
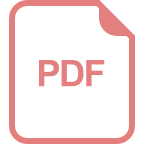
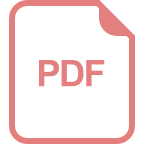
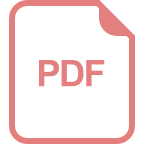












