怎样在PyQt5中解析LaTeX公式并实时更新?
时间: 2024-10-14 22:08:50 浏览: 42
在PyQt5中解析LaTeX公式并实现实时更新通常需要结合`qrcode`库、`matplotlib`库以及`sympy`库来完成。以下是一个简化的步骤:
1. 首先,你需要安装必要的库:
```
pip install PyQt5 matplotlib sympy qrcode
```
2. 导入所需的模块:
```python
from PyQt5.QtWidgets import QApplication, QLabel
from PyQt5.QtCore import pyqtSlot
import sympy
import matplotlib.pyplot as plt
from qrcode.image.svg import SvgPathImageWriter
```
3. 创建一个QLabel用于显示解析后的LaTeX:
```python
class LaTeXViewer(QLabel):
def __init__(self, parent=None):
super().__init__(parent)
self.text = QLabel(self)
self.text.setOpenExternalLinks(True)
@pyqtSlot(str)
def update_latex(self, latex_code):
self.latex_code = latex_code
self.update_display()
def update_display(self):
try:
formula = sympy.latex(self.latex_code)
svg_path = plt.imread(formula.replace("\\", ""), format="svg")
img = SvgPathImageWriter(svg_path).write_inline()
self.setText(img)
except Exception as e:
print(f"Error parsing LaTeX: {e}")
self.setText("Formula Error")
```
4. 在主窗口中实例化这个类,并连接解析函数到数据更改事件:
```python
app = QApplication([])
viewer = LaTeXViewer()
viewer.show()
# 假设你有一个字符串列表存储LaTeX公式
formulas = ["\int_{-\infty}^{\infty} e^{-x^2} dx", "\frac{d}{dx} \sin(x)"]
for formula in formulas:
viewer.update_latex(formula)
# 如果有实时更新的需求,你可以设置定时器或者信号槽关联来触发`update_latex`
# QTimer.singleShot(1000, lambda: viewer.update_latex(new_formula))
app.exec_()
```
阅读全文
相关推荐
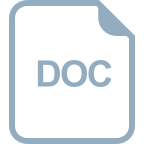
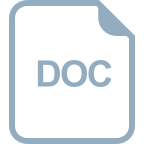
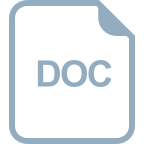

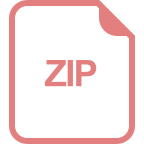
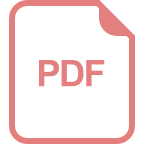
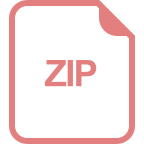
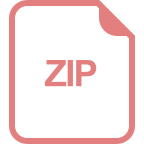
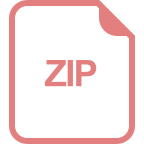
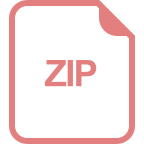
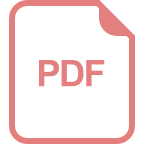
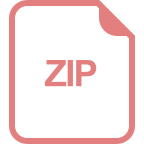
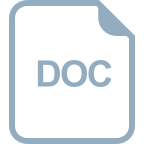
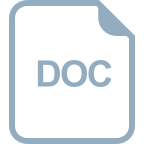
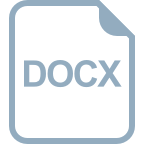