python rfc1867
时间: 2025-01-01 12:27:48 浏览: 5
### Python中实现RFC1867文件上传协议
RFC1867定义了通过HTTP POST方法使用`multipart/form-data`编码类型来上传文件的标准。在Python中,可以利用多种库和技术轻松实现这一标准。
#### 使用`requests`库进行文件上传
对于简单的文件上传需求,`requests`库提供了一个非常简便的方式来进行操作[^3]:
```python
import requests
url = 'http://example.com/upload'
files = {'upload_file': open('file.txt', 'rb')}
response = requests.post(url, files=files)
print(response.status_code)
```
这段代码展示了如何打开本地的一个文件并将其作为POST请求的一部分发送到指定URL。`requests`会自动处理`multipart/form-data`的细节部分。
#### 利用`urllib.request`与自定义边界字符串
如果希望更深入控制上传过程中的每一个环节,则可以通过构建原始HTTP消息体的方式来完成同样的工作。这里展示了一种基于`urllib.request`的方法,并指定了自己的边界字符串[^4]:
```python
from urllib import request
import mimetypes
boundary = '-- boundary-string --'
def encode_multipart_formdata(fields, files):
lines = []
for (key, value) in fields.items():
lines.append('--' + boundary)
lines.append(f'Content-Disposition: form-data; name="{key}"')
lines.append('')
lines.append(value)
for (name, filepath) in files:
with open(filepath, 'rb') as f:
data = f.read()
content_type = mimetypes.guess_type(filepath)[0] or 'application/octet-stream'
lines.append('--' + boundary)
lines.append(f'Content-Disposition: form-data; name="{name}"; filename="{filepath.split("/")[-1]}"')
lines.append(f'Content-Type: {content_type}')
lines.append('')
lines.append(data.decode())
lines.append('--' + boundary + '--')
lines.append('')
body = '\r\n'.join(lines).encode()
headers = {
"Content-Type": f'multipart/form-data; boundary={boundary}',
"Content-Length": str(len(body))
}
return body, headers
fields = {"field_name": "value"}
files = [("file_field", "/path/to/file")]
body, headers = encode_multipart_formdata(fields, files)
req = request.Request("http://example.com/post", method='POST', headers=headers, data=body)
resp = request.urlopen(req)
print(resp.read().decode())
```
此脚本手动构造了整个HTTP请求的消息体以及必要的头部信息,从而实现了对RFC1867的支持。
#### 结合`paramiko`用于安全传输
当涉及到敏感数据时,可能还需要考虑安全性方面的要求。此时可借助于像`paramiko`这样的SSH/SFTP库,在加密通道上执行文件上传任务[^2]:
```python
import paramiko
ssh_client = paramiko.SSHClient()
ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
ssh_client.connect(hostname='hostname', username='username', password='password')
sftp = ssh_client.open_sftp()
remote_path = '/remote/path/filename.ext'
local_path = './local/path/filename.ext'
sftp.put(local_path, remote_path)
finally:
sftp.close()
ssh_client.close()
```
上述例子说明了怎样建立一个SSH连接并通过它发起一次SFTP文件传送动作。
阅读全文
相关推荐











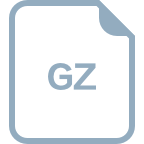
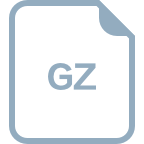
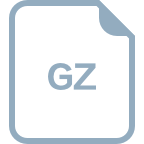
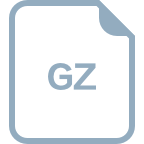




