std::map访问key
时间: 2024-03-15 14:39:46 浏览: 74
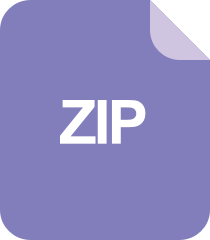
HashmapBenchmark:子弹哈希映射(btHashMap)和C++标准库哈希映射(std)之间的基准测试
在C++中,`std::map`是一种关联容器,它存储的元素是键值对(key-value pairs),其中键(key)是唯一的。你可以使用多种方式来访问键。
**1. 使用迭代器**
你可以使用迭代器来遍历`std::map`并访问键。以下是一个简单的示例:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
在上述代码中,`myMap.begin()`返回一个指向`myMap`中第一个元素的迭代器,`myMap.end()`返回一个指向`myMap`末尾的迭代器。通过迭代器,你可以访问键和对应的值。
**2. 使用 `at()` 方法**
另一种访问键的方式是使用 `at()` 方法。这个方法会检查键是否存在,如果键不存在,它会抛出一个`std::out_of_range`异常。因此,在使用 `at()` 方法时,你需要确保键在 `std::map` 中存在。以下是一个示例:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
int keyToFind = 4; // This key does not exist in the map
if (myMap.find(keyToFind) != myMap.end()) {
std::cout << "Key found: " << keyToFind << ", Value: " << myMap[keyToFind] << std::endl;
} else {
std::cout << "Key not found" << std::endl;
}
return 0;
}
```
在这个示例中,我们尝试查找一个不存在的键,并使用 `myMap.find()` 方法检查键是否存在。如果键存在,我们使用 `[]` 运算符来获取该键的值。如果键不存在,`myMap[keyToFind]` 会导致 `std::out_of_range` 异常。因此,使用 `at()` 方法时需要格外小心。
阅读全文
相关推荐
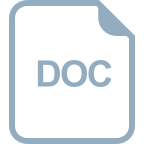
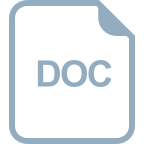















