golang docx 转pdf
时间: 2024-09-27 09:11:14 浏览: 84
在Go语言中,转换Word文档(docx)为PDF通常涉及到两个步骤:首先读取docx文件内容,然后将内容写入PDF。这需要使用第三方库,比如`github.com/360EntSecGroup-Skylar/excelize/v2`来处理DOCX,以及像`github.com/jung-kurt/gofpdf`这样的库来生成PDF。
以下是一个简单的示例流程:
1. 安装必要的库:
```bash
go get github.com/360EntSecGroup-Skylar/excelize/v2
go get github.com/jung-kurt/gofpdf
```
2. 使用`excelize`库读取DOCX:
```go
package main
import (
"github.com/360EntSecGroup-Skylar/excelize/v2"
)
func readDocx(file string) (*excelize.File, error) {
f := excelize.OpenFile(file)
return f, nil
}
```
3. 使用`gofpdf`库创建并写入PDF:
```go
package main
import (
"github.com/jung-kurt/gofpdf"
"path/filepath"
)
func writePdf(f *excelize.File, outputFilePath string) error {
pdf := gofpdf.New("P", "mm", "A4", "")
pdf.AddPage()
for _, sheet := range f.Sheets() {
rows := f.GetRows(sheet.Name())
for i, row := range rows {
for j, cell := range row {
pdf.Cell(i+1, j+1, fmt.Sprintf("%s", cell))
}
pdf.Ln(-1) // 换行
}
}
err := pdf.OutputFileAndClose(outputFilePath)
if err != nil {
return err
}
return nil
}
```
4. 将两者结合起来:
```go
func main() {
file, err := readDocx("input.docx")
if err != nil {
panic(err)
}
defer file.Close()
outputPath := "output.pdf"
err = writePdf(file, outputPath)
if err != nil {
panic(err)
}
fmt.Println("转换成功,结果保存在", outputPath)
}
```
阅读全文
相关推荐
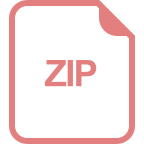
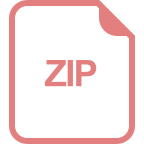
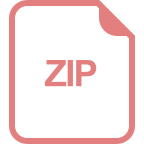


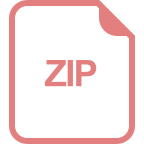
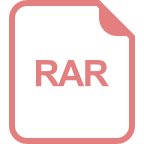
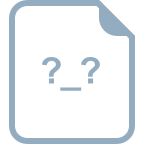
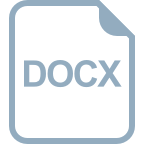



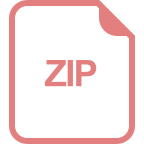
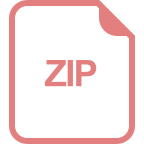
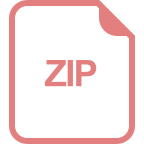
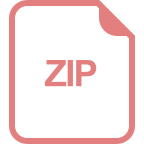
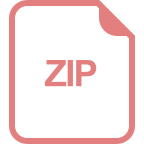
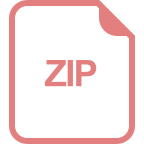