用pygame编写一个回合制小游戏
时间: 2023-09-11 11:13:18 浏览: 119
当然可以!下面是一个使用 Pygame 编写的简单回合制小游戏的示例代码:
```python
import pygame
import random
# 游戏初始化
pygame.init()
# 设置屏幕尺寸
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("回合制小游戏")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 定义角色类
class Character(pygame.sprite.Sprite):
def __init__(self, name):
super().__init__()
self.name = name
self.max_health = 100
self.current_health = self.max_health
self.attack_damage = random.randint(5, 15)
self.image = pygame.Surface([50, 50])
self.image.fill(WHITE)
self.rect = self.image.get_rect()
def attack(self, target):
target.current_health -= self.attack_damage
def update(self):
if self.current_health <= 0:
self.kill()
# 创建角色精灵组
all_characters = pygame.sprite.Group()
# 创建角色实例
player = Character("玩家")
enemy = Character("敌人")
# 将角色添加到精灵组
all_characters.add(player)
all_characters.add(enemy)
# 游戏主循环
running = True
clock = pygame.time.Clock()
while running:
# 检查事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 清除屏幕
screen.fill(BLACK)
# 更新角色状态
all_characters.update()
# 绘制角色
all_characters.draw(screen)
# 刷新屏幕
pygame.display.flip()
# 控制帧率
clock.tick(30)
# 游戏结束
pygame.quit()
```
这个示例代码创建了一个简单的回合制小游戏,其中有一个玩家角色和一个敌人角色。玩家和敌人都有一定的生命值和攻击力,每个回合玩家会攻击敌人,然后敌人会攻击玩家,直到其中一个角色的生命值归零为止。
你可以根据自己的需求修改代码,并添加其他功能、图像和音效等来使游戏更有趣。祝你编写游戏的过程愉快!
相关推荐
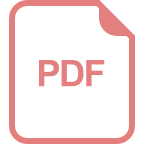
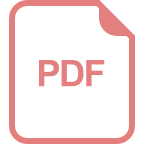














