具体如何实现,代码使用Arduino写
时间: 2024-10-16 07:05:10 浏览: 26
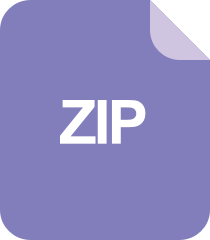
热-arduino蜂鸣器音乐代码
在Arduino平台上实现ESP8266作为Web服务器下载功能,你可以使用第三方库如`ESPAsyncWebServer`或`Ultralight Web Server`,它们简化了网络编程。以下是使用`ESPAsyncWebServer`的一个基本示例:
首先,你需要安装`ESPAsyncWebServer`库,可以在Arduino IDE的“管理库”里搜索并安装。安装完成后,可以按照以下步骤编写代码:
```cpp
#include <ESPAsyncWebServer.h>
#include "ESPAsyncTCP.h"
// ESP8266 Wi-Fi SSID和密码
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// 网站根目录和下载资源文件路径
const String rootPath = "/download/";
const String downloadFile = "file_to_download.zip";
AsyncWebServer server(80); // 默认HTTP服务器监听80端口
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
void loop() {
server.handleClient(); // 处理客户端请求
}
// 下载资源处理函数
AsyncWebResponse handleDownload(const AsyncWebRequest* request) {
if (request->url == rootPath + downloadFile) {
File fileToServe = SPIFFS.open(downloadFile, "r");
if (!fileToServe) {
return AsyncWebResponse(404, "File not found", "<h1>Error</h1>");
}
uint8_t contentLength = fileToServe.size();
String contentType = "application/zip"; // 设置合适的MIME类型
AsyncWebHeaderItem headers[] = {
{ "Content-Type", contentType.c_str() },
{ "Content-Length", String(contentLength).c_str() }
};
return AsyncWebResponse(200, "OK", headers, contentLength, &fileToServe);
} else {
return AsyncWebNotFound();
}
}
void setupServer() {
server.on("/", []() {
StaticFileHandler(rootPath, index.html);
});
server.on("/download/", handleDownload);
}
```
在`setup()`函数中,初始化WiFi连接和服务器。`loop()`函数用于持续监听客户端请求。`handleDownload()`函数处理下载请求,检查URL是否匹配,并从SPIFFS(嵌入式文件系统)读取文件内容。
最后,在`setupServer()`函数中注册路由规则,包括主页和下载页面。
记得替换`your_SSID`和`your_PASSWORD`为实际的Wi-Fi网络信息。运行程序后,连接到ESP8266的热点,通过浏览器访问`http://your_device_ip/download/file_to_download.zip`即可尝试下载。
阅读全文
相关推荐
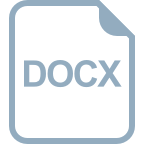
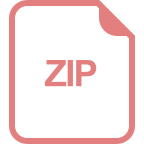
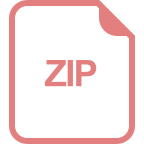
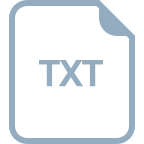
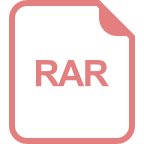
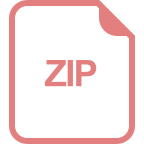
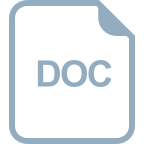
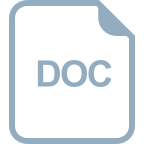
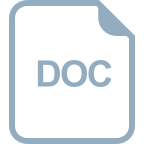
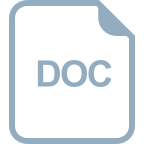
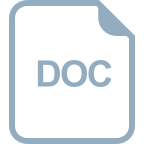
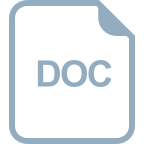
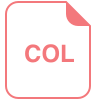
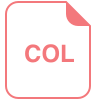


