如何在 Python 中实现通用的方法来读取和处理不同类型的文件(如文本文件、CSV、JSON 或二进制文件)?
时间: 2024-10-25 19:15:36 浏览: 31
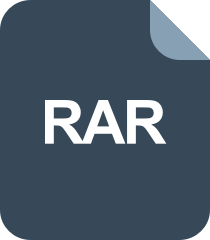
fp.rar_python_python 数据库_python读取数据_文件_读取文件
在Python中,为了实现能够处理多种文件类型(如文本文件、CSV、JSON和二进制文件)的通用方法,我们可以利用Python内置的模块和适配机制,例如`io`模块以及第三方库`csv`、`json`和`struct`等。下面是一个基础的框架:
1. **文本文件** (`.txt`): 使用内置的`open()`函数打开,然后逐行读取内容。
```python
def read_text_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
```
2. **CSV 文件** (`.csv`): 利用`csv`模块的`reader`函数。
```python
import csv
def read_csv_file(file_path):
with open(file_path, 'r', newline='') as file:
reader = csv.reader(file)
data = [row for row in reader]
return data
```
3. **JSON 文件** (`.json`): 使用`json`模块的`load()`函数。
```python
import json
def read_json_file(file_path):
with open(file_path, 'r') as file:
data = json.load(file)
return data
```
4. **二进制文件** (例如图片、音频或字节流): 可能需要根据具体的二进制结构使用`struct`、`numpy`或其他库解析。
```python
import struct
def read_binary_file(file_path, format_string='{}'):
with open(file_path, 'rb') as file:
bytes_data = file.read()
data = struct.unpack(format_string, bytes_data)
return data
```
**通用方法示例**:
```python
def generic_file_reader(file_path, handler_function):
if file_path.endswith('.txt'):
return handler_function(file_path)
elif file_path.endswith(('.csv', '.tsv')):
return handler_function(file_path, mode='r', delimiter=',' if file_path.endswith('.csv') else '\t')
elif file_path.endswith('.json'):
return handler_function(file_path, 'json')
elif file_path.endswith(('.bin', '.dat')):
return handler_function(file_path, 'binary', format_string)
else:
raise ValueError(f"Unsupported file type: {file_path}")
# 调用通用方法
data = generic_file_reader('example.txt', read_text_file)
```
阅读全文
相关推荐
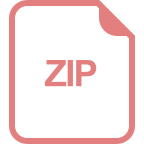
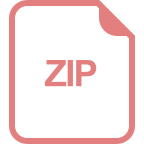
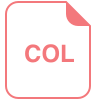
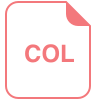
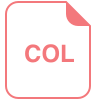
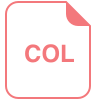
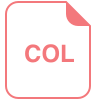
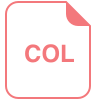








