go gin mqtt
时间: 2023-10-16 09:05:43 浏览: 152
这个问题是关于Go语言中的go.mod文件的错误。go.mod文件是用来管理Go模块依赖关系的配置文件。根据错误提示,当前目录或父目录中都没有找到go.mod文件。
在Go 1.16及更高版本中,使用go get命令来获取包已经不再支持在非模块化项目中使用了。取而代之的是使用go install命令来构建和安装命令,同时指定版本信息,比如'go install example.com/cmd@latest'。
要解决这个问题,你可以尝试以下几个步骤:
1. 确保你的项目目录下存在一个go.mod文件。如果不存在,可以使用以下命令初始化一个新的模块:
```
go mod init example.com/myproject
```
2. 确保你的项目目录是一个有效的Go模块。你可以通过运行以下命令来检查:
```
go env GOMOD
```
如果输出结果为空,那么你可能需要进入到正确的项目目录下再执行命令。
3. 如果你之前使用了go get命令来获取包,你可以尝试使用go install命令来替代,例如:
```
go install example.com/myproject@latest
```
注意要根据你的实际项目路径和版本信息来进行替换。
希望这些步骤能够帮助你解决问题。如果还有其他疑问,请随时提问。
相关问题
把这段代码转化为python代码(package service import ( "encoding/json" "errors" "fmt" "gin-syudy/api/device/req" "gin-syudy/define" "gin-syudy/models" "gin-syudy/mqtt" "gin-syudy/tools/resp" "gin-syudy/utils" mq "github.com/eclipse/paho.mqtt.golang" "github.com/gin-gonic/gin" "log" "net/http" "strconv" "time" ) // DeviceController 控制设备 // @BasePath /api/v1 // @Description 启动对应设备 // @Tags 启动设备 // @param identity query string false "Identity" // @param controllerId query string false "controllerId" // @param controlState query string false "controlState" // @Success 200 {object} resp.Response "{"code":200,"data":[...]}" // @Failure 502 {object} resp.Response "{"code":502,"data":[...]}" // @Router /api/v1/device/start [Post] func DeviceController(c *gin.Context) { device := new(models.DeviceBasic) write := new(mqtt.Write) device.Identity = c.Query("identity") id, _ := strconv.Atoi(c.Query("controllerId")) fmt.Println(id) state, _ := strconv.Atoi(c.Query("controllerState")) fmt.Println(state) write.Id = uint32(id) write.State = uint32(state) if device.Identity == "" { resp.RespFail(c, http.StatusBadGateway, errors.New("必填参数为空"), resp.FoundFail) return } deviceBasic := device.GetTopicByIdentity() subTopic := "Device/" + deviceBasic.ItemName + "/" + deviceBasic.BridgeName + "/control" + deviceBasic.Secret fmt.Println(subTopic) sendTopic := "Host/" + deviceBasic.ItemName + "/" + deviceBasic.BridgeName + "/control" + deviceBasic.Secret fmt.Println(sendTopic) dataChan := make(chan *mqtt.StartDataResp, 1) mqtt.SubscribeMessage(subTopic, func(client mq.Client, message mq.Message) { fmt.Printf("MESSAGE : %s\n", message.Payload()) fmt.Printf("TOPIC : %s\n", message.Topic()) subscribeStartData := new(mqtt.StartDataResp) err := json.Unmarshal(message.Payload(), &subscribeStartData) if err != nil { resp.RespFail(c, http.StatusBadGateway, err, "回调函数格式不正确") return } dataChan <- subscribeStartData }) startData := new(mqtt.StartData) startData.SampTime = time.Now().String() startData.CommandID = utils.GetUUid() startData.Write = write data, _ := json.Marshal(startData) err := mqtt.SendMessage(sendTopic, data) if err != nil { resp.RespFail(c, http.StatusBadGateway, err, resp.FoundFail) return } responseMessage := <-dataChan err, _ = mqtt.Unsubscribe(sendTopic) if err != nil { resp.RespFail(c, http.StatusBadGateway, err, "取消订阅失败") return } resp.RespOK(c, responseMessage, "控制成功") })
以下是将给定的 Go 代码翻译为 Python 的代码:
```python
import json
import logging
import time
from http import HTTPStatus
import paho.mqtt.client as mqtt
from flask import jsonify, request
from service.models import DeviceBasic
from service.mqtt import SendMessage, SubscribeMessage, Unsubscribe
from service.tools.resp import RespFail, RespOK
from service.utils import GetUUid
# DeviceController
# 控制设备
# @BasePath /api/v1
# @Description 启动对应设备
# @Tags 启动设备
# @param identity query string false "Identity"
# @param controllerId query string false "controllerId"
# @param controlState query string false "controlState"
# @Success 200 {object} resp.Response "{"code":200,"data":[...]}"
# @Failure 502 {object} resp.Response "{"code":502,"data":[...]}"
# @Router /api/v1/device/start [Post]
def DeviceController():
device = DeviceBasic()
device.identity = request.args.get('identity', '')
id = int(request.args.get('controllerId', 0))
logging.debug(f"id: {id}")
state = int(request.args.get('controllerState', 0))
logging.debug(f"state: {state}")
write = Write()
write.id = id
write.state = state
if not device.identity:
RespFail(HTTPStatus.BAD_GATEWAY, errors=["必填参数为空"])
return
deviceBasic = device.GetTopicByIdentity()
subTopic = f"Device/{deviceBasic.ItemName}/{deviceBasic.BridgeName}/control{deviceBasic.Secret}"
logging.debug(f"subTopic: {subTopic}")
sendTopic = f"Host/{deviceBasic.ItemName}/{deviceBasic.BridgeName}/control{deviceBasic.Secret}"
logging.debug(f"sendTopic: {sendTopic}")
dataChan = []
def on_message(client, userdata, message):
logging.debug(f"MESSAGE : {message.payload}")
logging.debug(f"TOPIC : {message.topic}")
subscribeStartData = mqtt.StartDataResp()
try:
subscribeStartData = json.loads(message.payload)
except json.JSONDecodeError as e:
RespFail(HTTPStatus.BAD_GATEWAY, errors=[f"回调函数格式不正确: {str(e)}"])
return
dataChan.append(subscribeStartData)
mqtt.SubscribeMessage(subTopic, on_message)
startData = mqtt.StartData()
startData.SampTime = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime())
startData.CommandID = GetUUid()
startData.Write = write
data = json.dumps(startData, default=lambda obj: obj.__dict__, ensure_ascii=False)
err = SendMessage(sendTopic, data.encode())
if err:
RespFail(HTTPStatus.BAD_GATEWAY, errors=[err])
return
responseMessage = dataChan[0]
err = Unsubscribe(sendTopic)
if err:
RespFail(HTTPStatus.BAD_GATEWAY, errors=[f"取消订阅失败: {err}"])
return
RespOK(responseMessage, message="控制成功")
```
go语言前景及适应范围
Go语言具有高效、简洁、并发、安全等特点,因此在云计算、分布式系统、大数据、网络编程等领域有着广泛的应用。目前,Go语言已经被许多互联网公司广泛应用,例如Google、Uber、Dropbox、Twitch、Netflix、Tencent等。
在云计算领域,Go语言被广泛用于开发容器技术(如Docker、Kubernetes等)、云函数(如AWS Lambda、阿里云函数计算等)等服务。
在分布式系统领域,Go语言的并发特性使其成为了一个非常适合开发分布式系统的语言,例如etcd、Consul等就是基于Go语言开发的。
在大数据处理领域,Go语言也有不少应用,例如InfluxDB、Grafana等都是基于Go语言开发的。
在网络编程领域,Go语言的高并发和高性能使其成为了开发网络服务器的不二之选,例如NATS、Mqtt、gin等都是基于Go语言开发的。
总之,Go语言的应用范围非常广泛,未来的前景也非常广阔。
阅读全文
相关推荐
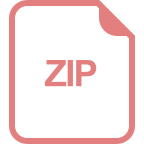
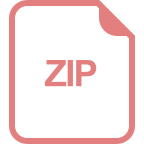
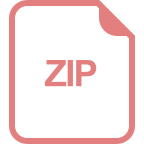
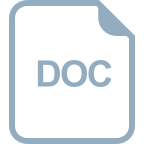
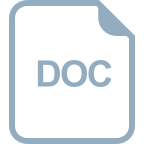
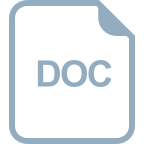
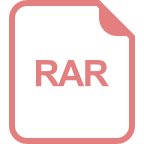
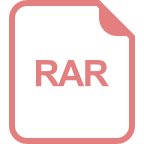
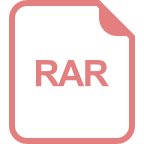
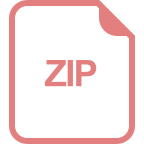
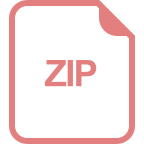