python协程读取文件
时间: 2023-08-27 21:19:42 浏览: 69
在Python中,可以使用协程(coroutine)来读取文件。协程是一种特殊的函数,它可以在执行过程中暂停和恢复。
在Python 3.5及以上的版本中,可以使用`asyncio`模块来实现协程。下面是一个使用协程读取文件的示例代码:
```python
import asyncio
async def read_file(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
# 模拟处理文件内容
await asyncio.sleep(1)
# 返回文件内容
return content
except FileNotFoundError:
print(f"File '{file_path}' not found.")
async def main():
file_path = 'path/to/your/file.txt'
content = await read_file(file_path)
print(content)
# 运行主协程
asyncio.run(main())
```
在这个例子中,首先定义了一个`read_file`协程函数,它接受一个文件路径作为参数,并使用`with open`语句打开文件并读取其中的内容。在模拟处理文件内容的过程中使用了`await asyncio.sleep(1)`来模拟耗时操作。
然后,在`main`函数中,调用`await read_file(file_path)`来调用协程函数,并使用`await`关键字等待协程函数执行完成。
最后,使用`asyncio.run(main())`来运行主协程。
这样就可以通过协程来异步读取文件内容了。注意,为了使用协程,需要在代码中使用`await`关键字来等待协程执行完成,并且在运行主协程时需要使用`asyncio.run()`方法。
相关推荐
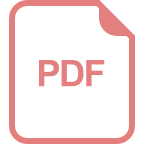
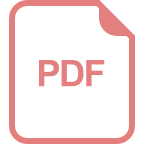
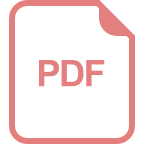














