用java语言创建学生类的泛型类, 包含姓名、成绩、出生日期属性。并通过传入类型实参建立学生类的多个实例,并打印出来
时间: 2024-12-19 14:22:10 浏览: 3
在Java中,我们可以创建一个泛型类来表示学生的通用信息,包括姓名、成绩和出生日期。这里是一个简单的例子:
```java
import java.util.Date;
public class GenericStudent<T> {
private String name;
private T score; // 使用泛型T来表示不同类型的成绩,如Integer或Double
private Date birthDate;
// 构造函数
public GenericStudent(String name, T score, Date birthDate) {
this.name = name;
this.score = score;
this.birthDate = birthDate;
}
// 获取方法
public String getName() {
return name;
}
public T getScore() {
return score;
}
public Date getBirthDate() {
return birthDate;
}
// 打印学生信息
@Override
public String toString() {
return "GenericStudent{" +
"name='" + name + '\'' +
", score=" + score +
", birthDate=" + birthDate +
'}';
}
}
// 创建具体的实例,例如学生的数学分数和出生日期
GenericStudent<Integer> mathStudent = new GenericStudent<>("张三", 95, new Date());
GenericStudent<Double> englishStudent = new GenericStudent<>("李四", 88.5, new Date());
// 打印学生
System.out.println(mathStudent); // 输出:GenericStudent{name='张三', score=95, birthDate=<date>}
System.out.println(englishStudent); // 输出:GenericStudent{name='李四', score=88.5, birthDate=<date>}
```
在这个示例中,`<T>`代表了泛型类型,我们可以在创建`GenericStudent`对象时指定具体的类型,如`Integer`或`Double`。这样可以使得类更具有灵活性,可以根据需要存储不同类型的数据。
阅读全文
相关推荐


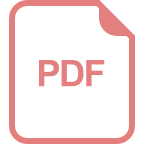
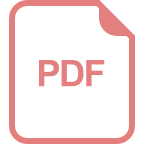

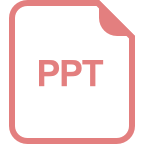
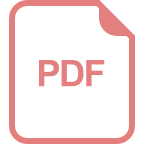
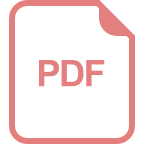
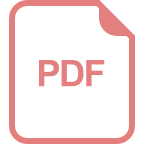
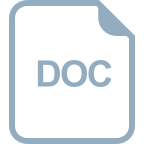
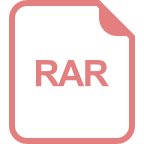
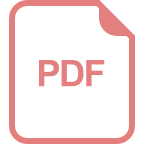
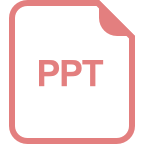
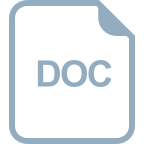
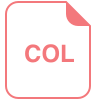
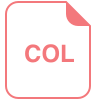
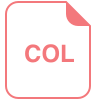
