python 对c++的构造函数,重载函数进行测试
时间: 2024-04-11 13:27:34 浏览: 12
当使用Python调用C++代码时,可以通过ctypes模块来调用C++的构造函数和重载函数。以下是一个示例:
假设有一个名为`example.cpp`的C++文件,其中包含一个`Person`类,具有构造函数和一个重载的`greet()`函数:
```cpp
#include <iostream>
#include <string>
class Person {
public:
Person(const std::string& name) : name_(name) {}
void greet() {
std::cout << "Hello, my name is " << name_ << std::endl;
}
void greet(const std::string& otherName) {
std::cout << "Hello, " << otherName << "! My name is " << name_ << std::endl;
}
private:
std::string name_;
};
```
首先,将C++代码编译为共享库(例如`example.so`)。
接下来,在Python中使用ctypes模块加载共享库,并测试构造函数和重载函数:
```python
import ctypes
# 加载共享库
lib = ctypes.CDLL('./example.so') # 根据实际的共享库路径进行修改
# 定义Person类
class Person:
def __init__(self, name):
# 调用C++的构造函数
lib.Person_new.argtypes = [ctypes.c_char_p]
lib.Person_new.restype = ctypes.c_void_p
self.obj = lib.Person_new(name.encode())
def greet(self, otherName=None):
if otherName is None:
# 调用C++的greet()函数
lib.Person_greet.argtypes = [ctypes.c_void_p]
lib.Person_greet.restype = None
lib.Person_greet(self.obj)
else:
# 调用C++的greet(const std::string& otherName)函数
lib.Person_greet_with_name.argtypes = [ctypes.c_void_p, ctypes.c_char_p]
lib.Person_greet_with_name.restype = None
lib.Person_greet_with_name(self.obj, otherName.encode())
# 创建Person对象并调用函数
p1 = Person("Alice")
p1.greet() # 输出:Hello, my name is Alice
p1.greet("Bob") # 输出:Hello, Bob! My name is Alice
```
在上述示例中,我们使用`ctypes`定义了Python中的`Person`类,并通过调用C++的构造函数和重载函数来创建对象和调用函数。
请注意,你需要根据实际的共享库路径进行修改。此外,确保C++代码在编译为共享库之前已经正确编写和编译。
相关推荐
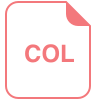












