python写一个动态的圣诞树
时间: 2023-12-25 21:30:01 浏览: 20
以下是一个用Python编写的动态圣诞树的例子:
```python
import time
def draw_tree(height):
stars = 1
for i in range(height):
print((' ' * (height - i)) + ('*' * stars))
stars += 2
print((' ' * height) + '|')
def draw_decorations():
decorations = ['*', 'o', '+', '^']
for i in range(10):
print(decorations[i % len(decorations)], end='')
time.sleep(0.5)
print()
def draw_christmas_tree(height):
draw_tree(height)
draw_decorations()
height = 5
draw_christmas_tree(height)
```
这段代码会先绘制一个指定高度的圣诞树,然后在树上绘制装饰物。装饰物会以星号、圆圈、加号和箭头的形式循环出现。你可以根据需要修改装饰物的种类和数量。
相关问题
python画一个动态圣诞树
以下是用Python画一个动态圣诞树的例子:
```python
import time
def draw_christmas_tree(height):
for i in range(height):
print((' ' * (height - i)) + ('*' * (2 * i + 1)))
print((' ' * height) + '|')
def animate_christmas_tree(height, duration):
for i in range(height):
print((' ' * (height - i)) + ('*' * (2 * i + 1)))
time.sleep(duration)
# 清除屏幕
print('\033c', end='')
print((' ' * height) + '|')
# 画一个静态的圣诞树
draw_christmas_tree(5)
# 画一个动态的圣诞树,每层之间间隔0.5秒
animate_christmas_tree(5, 0.5)
```
Python写一个圣诞树
在 Python 中,可以使用字符串来绘制圣诞树。例如,你可以使用多行字符串,其中每行都包含若干个空格和一个星号,来绘制圣诞树的形状:
```
tree = """
*
***
*****
*******
*********
***
"""
print(tree)
```
这将会打印出一颗五行的圣诞树,带有一个三行的圣诞树的树干。你可以通过修改字符串中的空格和星号的数量来调整圣诞树的大小和形状。
如果你想要更复杂的圣诞树,你可以使用循环和条件语句来动态生成圣诞树的各个部分。例如,你可以使用一个 for 循环来生成圣诞树的每一层,并使用一个 if 语句来控制是否在每一层的开头和结尾添加额外的空格,以便使圣诞树呈现出类似三角形的形状。
例如,你可以使用以下代码来生成一颗六层的圣诞树,其中每层都包含若干个星号,数量依次增加,并且每层的开头和结尾都有一个额外的空格:
```
for i in range(1, 7):
if i == 1:
print(" " * (6 - i) + "*")
elif i == 6:
print(" " * (6 - i) + "***")
else:
print(" " * (6 - i) + "*" * (2 * i - 1))
```
这将会打印出一颗
相关推荐
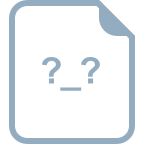
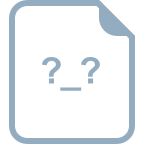


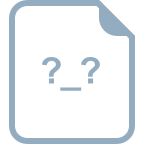
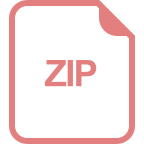
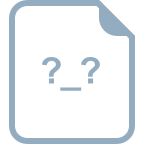
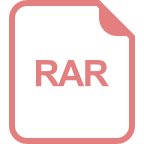
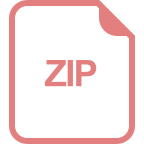