opengl里面画圆柱体
时间: 2024-12-31 10:44:20 浏览: 9
### 使用 OpenGL 绘制圆柱体
为了在 OpenGL 中绘制一个圆柱体,通常会采用三角形扇区 (Triangle Fan) 和三角带 (Triangle Strip) 的方式来构建几何形状。下面是一个完整的 C++ 示例程序,展示了如何创建并渲染一个简单的圆柱体。
#### 初始化顶点数据
首先定义用于描述圆柱表面的顶点数组:
```cpp
#include <GL/glut.h>
#include <cmath>
const int slices = 30;
GLfloat vertices[] = {
// Bottom cap vertices and texture coordinates...
};
void generateCylinderVertices(float radius, float height){
int index = 0;
// Add bottom circle center vertex with UV at origin.
vertices[index++] = 0.0f;
vertices[index++] = 0.0f;
vertices[index++] = -height/2.f;
for(int i=0;i<=slices;++i){ // Include the last point to close loop
double angleInRadians = M_PI * 2.0 * ((double)i/(double)slices);
// Side wall vertices
vertices[index++] = cos(angleInRadians)*radius;
vertices[index++] = sin(angleInRadians)*radius;
vertices[index++] = -height/2.f;
// Top cap vertices
vertices[index++] = cos(angleInRadians)*radius;
vertices[index++] = sin(angleInRadians)*radius;
vertices[index++] = height/2.f;
}
}
```
这段代码初始化了一个包含底部圆形中心以及围绕该中心分布的一系列边沿点的数据集[^1]。
#### 渲染函数实现
接着编写 `display` 函数,在其中调用上述生成好的顶点列表,并利用这些信息画出所需的图形结构:
```cpp
void display(){
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glBegin(GL_TRIANGLE_FAN);{
glVertex3fv(&vertices[0]); // Center of bottom cap
for(int i=3;i<3*(slices+2)+1;i+=3){
glVertex3fv(&vertices[i]);
}
} glEnd();
glBegin(GL_QUAD_STRIP);{
for(int i=0;i<(slices+1)*3;i+=3){
glVertex3fv(&vertices[i+3*slices+3]);
glVertex3fv(&vertices[i+3]);
}
}glEnd();
glBegin(GL_TRIANGLE_FAN);{
glVertex3fv(&vertices[(slices+1)*6]); // Center of top cap
for(int i=(slices+1)*3-3;i>=0;i-=3){
glVertex3fv(&vertices[i+3]);
}
}glEnd();
glutSwapBuffers();
}
```
这里分别使用了三个不同的绘图模式——`GL_TRIANGLE_FAN`, `GL_QUAD_STRIP` 来完成底面、侧面和顶面三部分的绘制工作。
#### 设置视窗环境
最后设置好窗口大小调整回调和其他必要的配置项即可启动应用程序:
```cpp
int main(int argc,char **argv){
glutInit(&argc, argv);
glutCreateWindow("OpenGL Cylinder");
glEnableClientState(GL_VERTEX_ARRAY);
glVertexPointer(3,GL_FLOAT,sizeof(GLfloat),vertices);
glutDisplayFunc(display);
glutReshapeFunc(resize);
init();
glutMainLoop();
return 0;
}
// Additional functions like resize() and init()
// should be implemented as needed by your application context.
```
此段代码片段提供了基本框架,具体细节可能依据实际需求有所不同。对于更复杂的场景,则需考虑加入纹理映射、光照计算等功能模块以增强视觉效果。
阅读全文
相关推荐



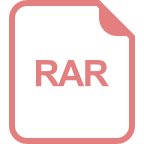







