python encrypt
时间: 2024-09-18 13:00:17 浏览: 98
在Python中,加密(encryption)主要是指通过编程将原始数据转换成看似无意义的密文,以保护其内容免受未经授权的访问。Python有许多流行的库可以实现加密,例如`pycryptodome`, `cryptography`, 和 `cryptography.fernet` 等。
其中,`cryptography` 是一个广泛使用的库,它提供了丰富的加密算法,包括对称加密(如AES、DES),非对称加密(如RSA、ECC)以及哈希函数等。例如,你可以使用AES(高级加密标准)对数据进行加密,示例代码如下:
```python
from cryptography.fernet import Fernet
# 生成随机密钥
key = Fernet.generate_key()
# 创建一个Fernet实例
cipher_suite = Fernet(key)
# 明文
plaintext = "This is a secret message"
# 对明文进行加密
encrypted_text = cipher_suite.encrypt(plaintext.encode())
print("Encrypted text:", encrypted_text)
```
解密时,只需使用相同的密钥即可还原原信息:
```python
# 解密密文
decrypted_text = cipher_suite.decrypt(encrypted_text).decode()
print("Decrypted text:", decrypted_text)
```
相关问题
Python encrypt
Python encrypt 是一个用于加密数据的Python库。根据提供的引用,有两种实现AES加密的方法可以参考。
方法1是使用pyAesCrypt库,代码如下:
```
import pyAesCrypt
def Encryption(input_file_path, output_file_path, key):
pyAesCrypt.encryptFile(input_file_path, output_file_path, key)
print("File has been encrypted")
def Decryption(input_file_path, output_file_path, key):
pyAesCrypt.decryptFile(input_file_path, output_file_path, key)
print("File has been decrypted")
```
方法2是使用cryptography库的Fernet模块,代码如下:
```
from cryptography.fernet import Fernet
def encrypt(filename, key):
fernet = Fernet(key)
with open(filename, 'rb') as file:
original = file.read()
encrypted = fernet.encrypt(original)
with open(filename, 'wb') as enc_file:
enc_file.write(encrypted)
key = Fernet.generate_key()
filename = "file.txt"
encrypt(filename, key)
```
以上两种方法都可以用于对文件进行加密和解密操作。你可以根据需求选择适合的方法来保护你的数据。
python encrypt.encrypt
在Python中进行加密操作通常需要使用加密算法库,比如`cryptography`库。这个库提供了各种常见的加密算法,可以用来进行数据加密。
首先,你需要安装`cryptography`库。你可以使用以下命令通过pip安装:
```
pip install cryptography
```
一旦安装完成,你可以使用以下代码进行加密操作:
```python
from cryptography.fernet import Fernet
def encrypt(message, key):
f = Fernet(key)
encrypted_message = f.encrypt(message)
return encrypted_message
# 生成一个新的密钥
key = Fernet.generate_key()
# 要加密的消息
message = b"Hello, World!"
# 进行加密
encrypted_message = encrypt(message, key)
print("加密后的消息:", encrypted_message)
```
这个例子中使用了Fernet对称加密算法。你可以使用`Fernet.generate_key()`生成一个随机的密钥,然后使用`encrypt()`函数对消息进行加密。最后打印出加密后的消息。
请注意,这只是一个简单的示例,实际使用时你可能需要更复杂的操作和更安全的密钥管理方法。
阅读全文
相关推荐
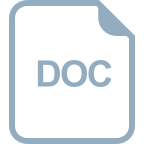
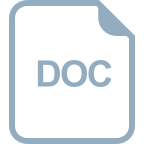
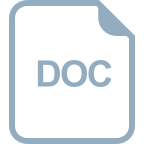
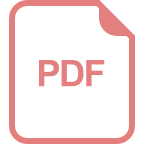
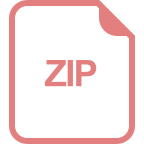


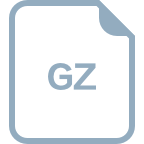
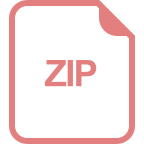
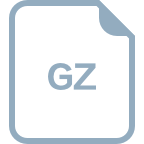
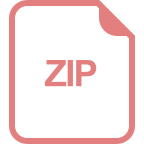
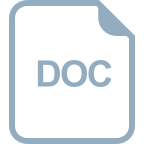
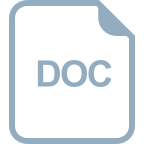
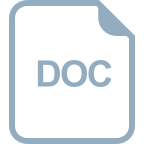
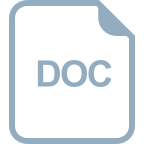
