delete1:function (id){ this.list=this.list.filter((item)=>item.id!==id) }这个是什么意思,为什么我用这个方法没结果出来,帮我改一下
时间: 2024-09-22 20:09:06 浏览: 40
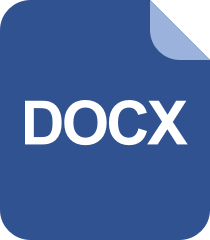
React框架教程.docx
这段代码是在JavaScript中定义的一个名为`delete1`的方法,它接收一个参数`id`。其作用是从数组`this.list`中过滤出所有不等于给定`id`的元素,然后将处理后的数组赋值回`this.list`。这种方法假设`this.list`是一个包含对象的数组,每个对象都有一个`id`属性。
如果使用这个方法后没有得到预期的结果,可能的原因有:
1. `this.list`不是一个数组,或者根本不存在,导致无法执行滤操作。
2. `id`参数传递错误,可能是null、undefined、空字符串或者与其他项的id相等。
3. 数组中的对象没有`id`属性,或者该属性不是可比较的类型(比如是对象而非基本数据类型)。
为了解决这个问题,你需要确保`this.list`存在并且是个数组,`id`参数已正确传递,并且列表中的对象确实有一个`id`属性。这里是修改后的代码示例:
```javascript
if (Array.isArray(this.list) && typeof id === 'number') { // 假设id是数字类型
this.list = this.list.filter(item => item.id !== id);
} else {
console.error("Invalid list or id type"); // 如果条件不满足,输出错误信息
}
```
这样可以先检查list和id的类型是否正确,再执行过滤操作。
阅读全文
相关推荐
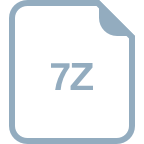
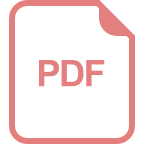















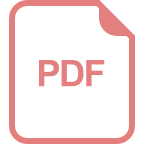