Java代码新建一个顺序栈,栈中每个元素的属性有id和name,实现入栈和出栈等操作
时间: 2024-10-22 19:05:12 浏览: 25
在Java中,我们可以创建一个简单的顺序栈(基于数组实现),其中存储`Element`类型的对象,每个`Element`包含'id'和'name'两个属性。下面是一个基本的实现:
```java
public class SimpleStack {
private int top;
private Element[] stack; // 使用数组存储元素
// 初始化栈的容量
public SimpleStack(int capacity) {
this.stack = new Element[capacity];
this.top = -1;
}
// 入栈(push)操作
public void push(Element item) {
if (top < stack.length - 1) { // 防止栈满
stack[++top] = item;
} else {
System.out.println("Stack is full");
}
}
// 出栈(pop)操作
public Element pop() {
if (top >= 0) { // 只有当栈非空时才能出栈
return stack[top--]; // 返回并移除栈顶元素
} else {
System.out.println("Stack is empty");
return null; // 或者抛出异常,视情况而定
}
}
// 查看栈顶元素(peek)
public Element peek() {
if (top >= 0) {
return stack[top];
} else {
System.out.println("Stack is empty");
return null; // 同样处理空栈的情况
}
}
// 判断栈是否为空
public boolean isEmpty() {
return top == -1;
}
// 获取栈的大小
public int size() {
return top + 1;
}
}
// 定义Element类
class Element {
private int id;
private String name;
public Element(int id, String name) {
this.id = id;
this.name = name;
}
// getters and setters for id and name
}
```
阅读全文
相关推荐
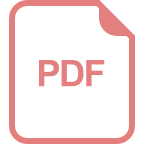
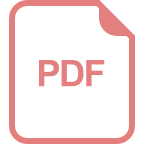
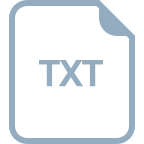
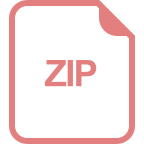
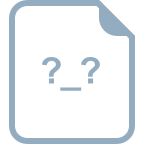
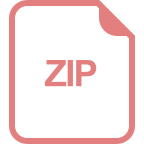
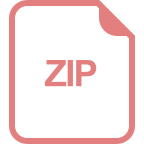
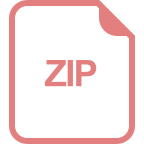
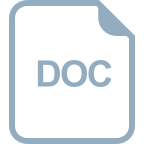
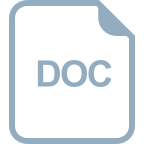
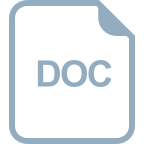
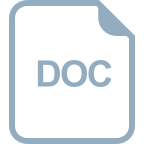
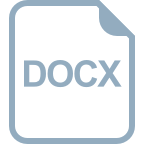