javaweb上传图片到数据库
时间: 2023-11-29 09:45:19 浏览: 164
在JavaWeb中上传图片到数据库需要进行以下几个步骤:
1.在数据库中创建表,其中包含一个用于存储图片的BLOB类型的字段。
2.在JSP页面中添加一个表单,用于上传图片。
3.在Servlet中获取表单数据,包括上传的图片。
4.将图片转换为字节数组,并将其存储到数据库中。
5.在JSP页面中显示从数据库中检索到的图片。
以下是一个简单的示例代码:
1.创建数据库表
```sql
CREATE TABLE `image` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) DEFAULT NULL,
`data` longblob,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
2.添加上传表单
```html
<form action="upload" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="Upload">
</form>
```
3.处理上传请求
```java
@WebServlet("/upload")
@MultipartConfig
public class UploadServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
InputStream inputStream = null;
OutputStream outputStream = null;
Connection connection = null;
PreparedStatement statement = null;
try {
Part filePart = request.getPart("file");
if (filePart != null) {
inputStream = filePart.getInputStream();
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
statement = connection.prepareStatement("INSERT INTO image (name, data) values (?, ?)");
statement.setString(1, filePart.getName());
statement.setBlob(2, inputStream);
statement.executeUpdate();
}
response.sendRedirect("index.jsp");
} catch (Exception e) {
e.printStackTrace();
} finally {
if (inputStream != null) {
inputStream.close();
}
if (outputStream != null) {
outputStream.close();
}
if (statement != null) {
statement.close();
}
if (connection != null) {
connection.close();
}
}
}
}
```
4.从数据库中检索图片并显示
```java
@WebServlet("/image")
public class ImageServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Connection connection = null;
PreparedStatement statement = null;
ResultSet resultSet = null;
OutputStream outputStream = null;
try {
int id = Integer.parseInt(request.getParameter("id"));
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
statement = connection.prepareStatement("SELECT * FROM image WHERE id = ?");
statement.setInt(1, id);
resultSet = statement.executeQuery();
if (resultSet.next()) {
Blob blob = resultSet.getBlob("data");
byte[] bytes = blob.getBytes(1, (int) blob.length());
response.setContentType("image/jpeg");
outputStream = response.getOutputStream();
outputStream.write(bytes);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (outputStream != null) {
outputStream.close();
}
if (resultSet != null) {
resultSet.close();
}
if (statement != null) {
statement.close();
}
if (connection != null) {
connection.close();
}
}
}
}
```
阅读全文
相关推荐

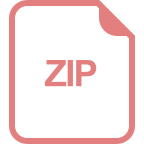
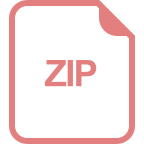
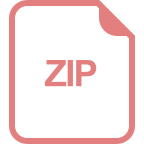


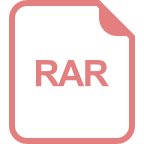
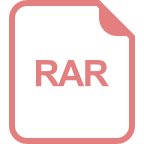
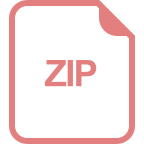
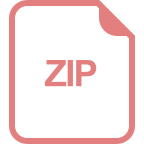
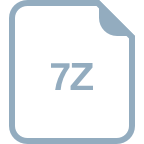
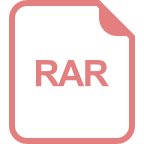
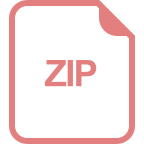
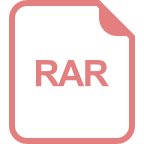
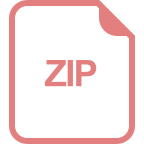
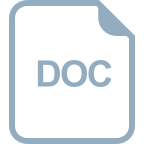