建立两条长度为n的单链表并输出c语言
时间: 2024-10-26 19:04:04 浏览: 10
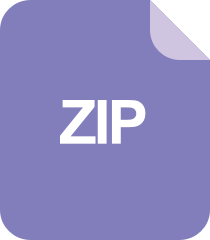
双向链表实现贪吃蛇游戏(C语言版)
在 C 语言中,你可以这样做:
首先,定义一个链表节点结构体 `ListNode`:
```c
struct ListNode {
int data; // 节点的数据部分
struct ListNode* next; // 指向下一个节点的指针
};
```
接着,创建链表的函数 `createLinkedList`:
```c
struct ListNode* createLinkedList(int n, int val) {
if (n <= 0) {
return NULL;
}
struct ListNode* head = (struct ListNode*)malloc(sizeof(struct ListNode));
head->data = val;
head->next = createLinkedList(n - 1, val); // 递归调用,生成剩余节点
return head;
}
```
分别创建两条长度为 n 的链表,比如 `listA` 和 `listB`:
```c
int n = 5; // 链表长度
struct ListNode* listA = createLinkedList(n, 1);
struct ListNode* listB = createLinkedList(n, 2);
```
最后,遍历链表并输出其内容:
```c
void printLinkedList(struct ListNode* node, char name[]) {
while (node != NULL) {
printf("%s: %d ->", name, node->data);
node = node->next;
}
printf("NULL\n");
}
// 输出链表
printLinkedList(listA, "List A");
printLinkedList(listB, "List B");
```
这将创建两条长度为 n 的单链表,并打印它们各自的数据。
阅读全文
相关推荐
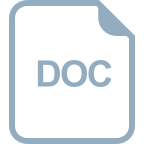
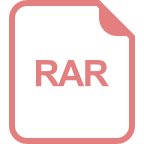
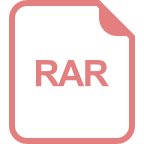
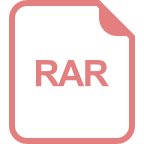
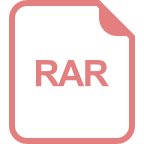
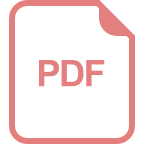
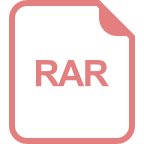
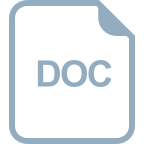
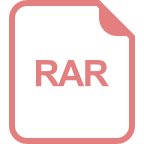
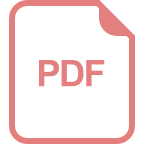
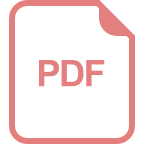
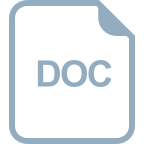
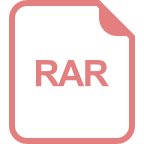
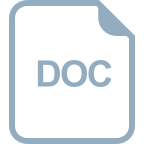
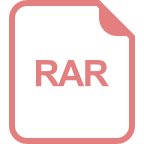
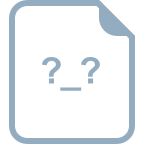