设计一个时间类time,可以设置时间并 进行时间的加减运算,在其中添加友元函数以完成时间的输出,最后编写主函数测试。.
时间: 2024-10-17 21:14:54 浏览: 27
设计一个名为`Time`的时间类,首先需要包含小时、分钟和秒作为成员变量。我们可以使用结构体或类来实现这个功能,这里假设我们使用类:
```cpp
#include <iostream>
class Time {
private:
int hours;
int minutes;
int seconds;
public:
// 构造函数初始化时间和友元函数声明
Time(int h = 0, int m = 0, int s = 0) : hours(h), minutes(m), seconds(s) {}
// 友元函数用于设置时间
friend void setTime(Time &t, int h, int m, int s);
// 友元函数用于获取时间字符串表示
friend std::string getTimeString(const Time &t);
// 时间的加减运算
Time operator+(const Time &other) const;
Time operator-(const Time &other) const;
// 设置时间
void setHours(int hours) { this->hours = hours; }
void setMinutes(int minutes) { this->minutes = minutes; }
void setSeconds(int seconds) { this->seconds = seconds; }
// 获取当前时间
int getHours() const { return hours; }
int getMinutes() const { return minutes; }
int getSeconds() const { return seconds; }
};
// 实现友元函数
void setTime(Time &t, int h, int m, int s) {
t.hours = h;
t.minutes = m;
t.seconds = s;
}
std::string getTimeString(const Time &t) {
return std::to_string(t.getHours()) + ":" + std::to_string(t.getMinutes()) + ":" + std::to_string(t.getSeconds());
}
// 时间的加减运算实现
Time Time::operator+(const Time &other) const {
int total_hours = hours + other.hours;
int total_minutes = minutes + other.minutes;
int total_seconds = seconds + other.seconds;
while (total_minutes >= 60) {
total_hours += total_minutes / 60;
total_minutes %= 60;
}
while (total_seconds >= 60) {
total_minutes += total_seconds / 60;
total_seconds %= 60;
}
return Time(total_hours, total_minutes, total_seconds);
}
Time Time::operator-(const Time &other) const {
int result_hours = hours - other.hours;
int result_minutes = minutes - other.minutes;
int result_seconds = seconds - other.seconds;
if (result_seconds < 0) {
result_minutes--;
result_seconds += 60;
}
if (result_minutes < 0 && result_hours == 0) {
result_hours--;
result_minutes += 60;
}
return Time(result_hours, result_minutes, result_seconds);
}
int main() {
Time time1(10, 30, 45);
Time time2(2, 15, 0);
std::cout << "Time 1: " << getTimeString(time1) << std::endl;
std::cout << "Time 2: " << getTimeString(time2) << std::endl;
Time combined_time = time1 + time2;
std::cout << "Combined time: " << getTimeString(combined_time) << std::endl;
Time difference = time1 - time2;
std::cout << "Difference: " << getTimeString(difference) << std::endl;
return 0;
}
```
在这个例子中,`Time`类包含了基本的时间操作,如设置和获取小时、分钟和秒,并提供了友元函数以方便输出时间和进行加减运算。在`main`函数中,我们创建了两个`Time`对象并进行了简单的操作演示。
阅读全文
相关推荐
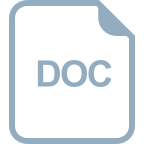
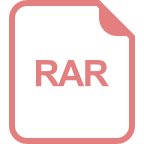
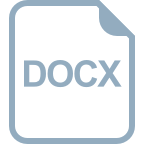
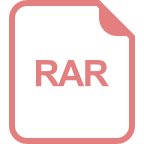
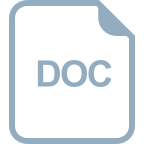
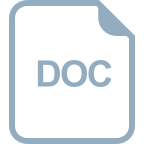
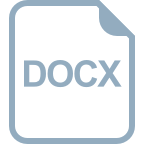
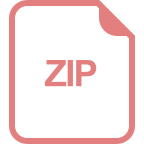
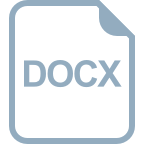
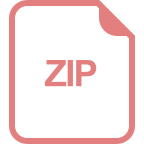
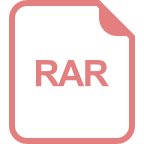
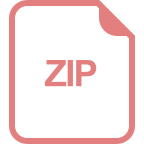
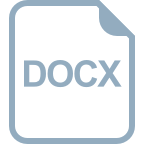
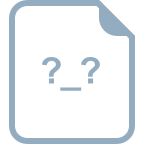