如何使用C#编写一个HTTP GET请求的方法,该方法接受一个集合作为参数并返回接口的数据?
时间: 2024-10-25 19:18:43 浏览: 28
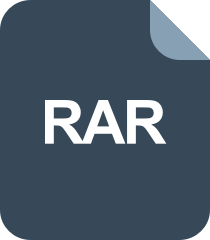
完整的接口调用实例,包括请求类、返回实体类、接口调用、接口处理文件
在C#中,你可以使用HttpClient类来发送HTTP GET请求。首先,你需要添加System.Net.Http命名空间。以下是一个示例方法,它接受一个字符串类型的集合作为参数,并返回接口数据:
```csharp
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Threading.Tasks;
public class HttpRequestService
{
private readonly HttpClient _httpClient;
public HttpRequestService()
{
_httpClient = new HttpClient();
}
// 这里假设你要从URL 'baseUri' 获取数据,并且接口的参数在查询字符串中传递
public async Task<IEnumerable<string>> GetDataAsync(IEnumerable<KeyValuePair<string, string>> queryParams)
{
if (queryParams == null || queryParams.Count() == 0)
throw new ArgumentException("Query parameters cannot be empty.");
string query = string.Join("&", queryParams.Select(pair => $"{pair.Key}={pair.Value}"));
var response = await _httpClient.GetAsync($"{baseUri}?{query}");
if (!response.IsSuccessStatusCode)
{
throw new Exception($"Failed to fetch data with status code {response.StatusCode}");
}
string responseBody = await response.Content.ReadAsStringAsync();
return Newtonsoft.Json.DeserializeObject<IEnumerable<string>>(responseBody);
}
}
// 调用方法的方式
List<KeyValuePair<string, string>> paramsList = new List<KeyValuePair<string, string>> { new KeyValuePair<string, string>("param1", "value1"), new KeyValuePair<string, string>("param2", "value2") };
HttpRequestService requestService = new HttpRequestService();
Task<IEnumerable<string>> resultTask = requestService.GetDataAsync(paramsList);
try
{
IEnumerable<string> data = await resultTask;
Console.WriteLine($"Received data: {data}");
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
```
在这个例子中,`GetDataAsync`方法接收一个`KeyValuePair`列表作为参数,构建查询字符串,然后发送GET请求到指定的URL。如果请求成功,它会解析返回的JSON内容并将其转换回一组字符串。
阅读全文
相关推荐
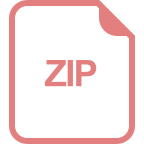
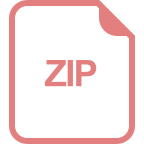















