c++编写文件保险箱
时间: 2024-09-21 16:06:14 浏览: 43
在C++中,你可以使用文件流(fstream)库来创建一个简单的“文件保险箱”概念,即用于存储和管理数据的容器。这个保险箱可以包含一系列的功能,如读取、写入、追加以及删除文件中的内容。下面是一个基础的示例,展示了如何创建一个基本的文本文件保险箱:
```cpp
#include <iostream>
#include <fstream>
#include <string>
// 文件保险箱类
class FileSafeBox {
public:
// 构造函数
FileSafeBox(const std::string& fileName) : file(fileName) {
if (!file.is_open()) {
std::cout << "Failed to open file: " << fileName << std::endl;
return;
}
std::cout << "Opened file: " << fileName << std::endl;
}
// 写入数据
void writeData(const std::string& data) {
file << data << "\n";
}
// 读取数据
std::string readData() {
std::string line;
getline(file, line);
return line; // 这里假设每行都是独立的数据
}
// 关闭文件
~FileSafeBox() {
file.close();
std::cout << "Closed file: " << fileName << std::endl;
}
private:
std::ifstream file; // 输入流用于读取
std::ofstream file; // 输出流用于写入
};
int main() {
FileSafeBox box("data.txt");
box.writeData("Hello, this is a secret message.");
std::string message = box.readData();
std::cout << "Read data: " << message << std::endl;
return 0;
}
```
在这个例子中,`FileSafeBox` 类代表了保险箱,它有构造函数打开文件,提供写入和读取数据的方法,并在析构函数关闭文件。用户可以在`main()` 函数中操作这个保险箱。
阅读全文
相关推荐
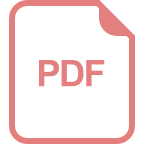
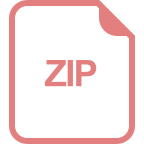
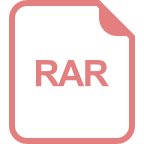
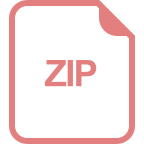
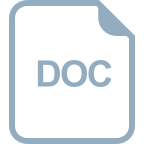
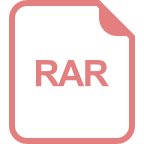
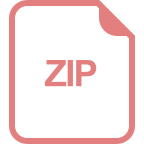
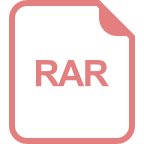
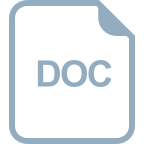
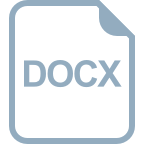
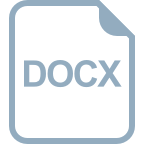
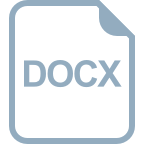
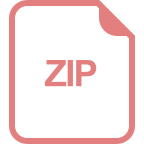