vue3组合式API实现视频播放,允许拖动进度条,打点评论,显示弹幕,进度条显示打点点击跳转进度,具体实现代码
时间: 2024-04-15 22:30:56 浏览: 139
要使用Vue3的组合式API实现视频播放、允许拖动进度条、打点评论、显示弹幕以及进度条上显示打点并点击跳转进度,你可以按照以下步骤进行:
1. 安装依赖:首先,你需要安装Vue3和其他必要的依赖。
```bash
npm install vue@next video.js
```
2. 创建视频播放器组件:使用Vue3的组合式API创建一个视频播放器组件。
```vue
<template>
<div>
<video ref="videoRef" class="video-player"></video>
<div class="progress-bar" @click="seek">
<div class="progress" :style="{ width: progress + '%' }"></div>
<div
v-for="marker in markers"
class="marker"
:style="{ left: marker.time * 100 + '%' }"
@click="jumpToMarker(marker)"
></div>
</div>
<div class="danmu-layer">
<!-- 这里显示弹幕 -->
</div>
</div>
</template>
<script>
import { ref, onMounted, reactive } from 'vue';
import videojs from 'video.js';
export default {
setup() {
const videoRef = ref(null);
const progress = ref(0);
const markers = reactive([
// 打点评论弹幕信息
{ time: 0.1, comment: '这是一个打点评论弹幕' },
// ...
]);
onMounted(() => {
const videoPlayer = videojs(videoRef.value, {}, () => {
// 视频加载完成后的回调函数
videoPlayer.on('timeupdate', () => {
const currentTime = videoPlayer.currentTime();
const duration = videoPlayer.duration();
progress.value = (currentTime / duration) * 100;
});
});
});
const seek = (event) => {
const progressBar = event.currentTarget;
const rect = progressBar.getBoundingClientRect();
const offsetX = event.clientX - rect.left;
const progress = offsetX / rect.width;
const duration = videoRef.value.duration;
const currentTime = duration * progress;
videoRef.value.currentTime = currentTime;
};
const jumpToMarker = (marker) => {
videoRef.value.currentTime = marker.time;
};
return {
videoRef,
progress,
markers,
seek,
jumpToMarker,
};
},
};
</script>
<style scoped>
.video-player {
width: 100%;
height: auto;
}
.progress-bar {
width: 100%;
height: 10px;
background-color: #ddd;
position: relative;
cursor: pointer;
}
.progress {
height: 100%;
background-color: #333;
width: 0;
}
.marker {
position: absolute;
top: -5px;
width: 5px;
height: 20px;
background-color: red;
}
.danmu-layer {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
</style>
```
在这个示例中,我们使用了Vue3的组合式API来创建视频播放器组件。通过在`setup()`函数中定义响应式数据和方法,我们可以轻松地实现视频播放、进度条拖动、打点评论弹幕和点击跳转进度的功能。
在`onMounted()`生命周期钩子中,我们使用`videojs`库来初始化视频播放器并监听时间更新事件,以更新进度条的位置。
在模板中,我们使用`ref`来引用视频元素和进度条元素,并使用相应的事件处理函数来实现拖动进度条和点击打点跳转进度。
请注意,这只是一个简单的示例代码,你可能需要根据实际需求进行定制和扩展。例如,你可以添加弹幕显示逻辑、自定义样式等。
记得安装`video.js`库,并根据自己的需求修改代码中的视频源、打点评论弹幕信息等。
阅读全文
相关推荐
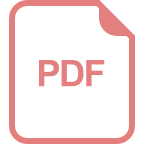
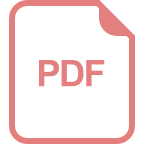
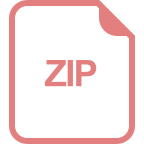

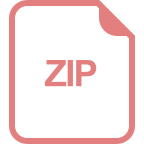

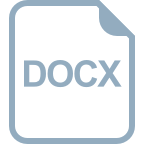
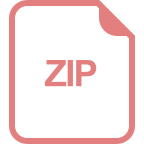
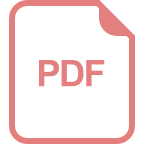
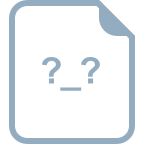
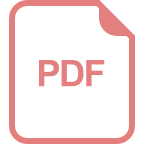
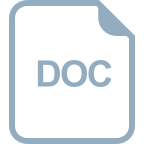
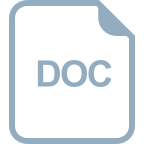