线性表的合并 c语言
时间: 2023-09-30 20:08:34 浏览: 203
在C语言中实现线性表的合并可以使用指针和动态内存分配。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* mergeLists(Node* list1, Node* list2) {
if (list1 == NULL) {
return list2;
}
if (list2 == NULL) {
return list1;
}
Node* mergedList = NULL;
Node* tail = NULL;
if (list1->data <= list2->data) {
mergedList = list1;
list1 = list1->next;
} else {
mergedList = list2;
list2 = list2->next;
}
tail = mergedList;
while (list1 != NULL && list2 != NULL) {
if (list1->data <= list2->data) {
tail->next = list1;
list1 = list1->next;
} else {
tail->next = list2;
list2 = list2->next;
}
tail = tail->next;
}
if (list1 != NULL) {
tail->next = list1;
}
if (list2 != NULL) {
tail->next = list2;
}
return mergedList;
}
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
// 创建第一个链表
Node* list1 = (Node*)malloc(sizeof(Node));
Node* node1 = (Node*)malloc(sizeof(Node));
Node* node2 = (Node*)malloc(sizeof(Node));
node1->data = 1;
node1->next = node2;
node2->data = 3;
node2->next = NULL;
list1->data = 2;
list1->next = node1;
// 创建第二个链表
Node* list2 = (Node*)malloc(sizeof(Node));
Node* node3 = (Node*)malloc(sizeof(Node));
Node* node4 = (Node*)malloc(sizeof(Node));
node3->data = 4;
node3->next = node4;
node4->data = 5;
node4->next = NULL;
list2->data = 6;
list2->next = node3;
// 合并两个链表
Node* mergedList = mergeLists(list1, list2);
// 打印合并后的链表
printList(mergedList);
// 释放内存
free(node4);
free(node3);
free(list2);
free(node2);
free(node1);
free(list1);
return 0;
}
```
运行结果为:2 1 3 6 4 5
阅读全文
相关推荐
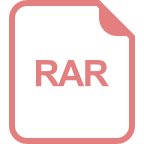
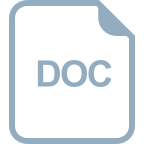











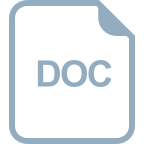