写一个基于Python的简易股票交易模拟系统
时间: 2024-11-27 07:12:39 浏览: 12
一个简单的Python股票交易模拟系统可以包括以下几个关键部分:
1. **股票类** (Stock): 创建一个表示股票的对象,包含属性如名称、当前价格、购买价格等,并提供买入(Buy)和卖出(Sell)方法。
```python
class Stock:
def __init__(self, name, price):
self.name = name
self.price = price
self.purchase_price = None
def buy(self, quantity, current_price):
if not self.purchase_price:
self.purchase_price = current_price
else:
print(f"Not enough funds for {quantity} shares of {self.name}")
# 更新购买记录
def sell(self, quantity, current_price):
if self.purchase_price and self.balance >= quantity * current_price:
profit = (current_price - self.purchase_price) * quantity
self.purchase_price = None
print(f"Sold {quantity} shares of {self.name}, profit: {profit}")
else:
print("Insufficient holdings or insufficient balance")
@property
def balance(self):
return self.price * (self.purchase_price or 0)
```
2. **账户类** (Account): 管理用户的投资组合,存储股票对象和可用资金。
```python
class Account:
def __init__(self, initial_funds):
self.funds = initial_funds
self.portfolio = {}
def add_stock(self, stock, quantity):
self.portfolio[stock] = quantity
def trade(self, stock_name, action, quantity, current_price):
if stock_name in self.portfolio:
stock = self.portfolio[stock_name]
stock[action](quantity, current_price)
else:
print(f"No {stock_name} in portfolio.")
```
3. **主程序** (Main):
```python
def main():
stock = Stock('AAPL', 150)
account = Account(10000)
account.add_stock(stock, 100)
account.trade('AAPL', 'buy', 50, stock.price)
# 更改实时股价并进行买卖操作...
if __name__ == "__main__":
main()
```
阅读全文
相关推荐





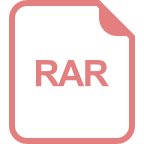












