python怎么对压缩包加密
时间: 2024-09-14 11:07:11 浏览: 42
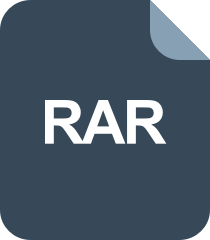
Python破解zip加密压缩包

在Python中,你可以使用`zipfile`模块配合`cryptography`库来加密压缩文件。以下是一个简单的步骤:
首先,确保已经安装了`cryptography`库,如果没有,可以使用pip安装:
```bash
pip install cryptography
```
然后,你可以编写一个函数来创建加密的ZIP文件:
```python
from zipfile import ZipFile
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
import os
def encrypt_zip(file_path, output_path, password):
# 初始化AES密钥和初始化向量
key = os.urandom(16) # 密钥长度必须为16字节(AES-128)
cipher = AES.new(key, AES.MODE_CBC)
# 加密密码并将其添加到加密文件头部作为前导
iv = cipher.iv
encrypted_key = cipher.encrypt(pad(password.encode(), AES.block_size))
# 创建一个ZipFile对象,并打开输出文件
with ZipFile(output_path, 'w', compression=zipfile.ZIP_DEFLATED) as zipped_file:
# 对每个文件进行加密处理
for root, dirs, files in os.walk(file_path):
for file in files:
source_file = os.path.join(root, file)
with open(source_file, 'rb') as src_file:
content = src_file.read()
# 加密内容
encrypted_content = cipher.encrypt(pad(content, AES.block_size))
# 将加密后的文件数据写入Zip
zipped_file.writestr(source_file, encrypted_content)
# 写入加密的密钥和初始化向量到一个单独的文件(如.key文件),用于后续解密
with open('key.txt', 'wb') as key_file:
key_file.write(iv + encrypted_key)
# 使用示例
encrypt_zip('/path/to/source/files', '/path/to/output.zip', 'my_secret_password')
```
在这个例子中,我们首先生成一个随机的AES密钥,然后将密码进行填充加密。在创建ZipFile对象时,我们将文件内容加密后再写入。最后,我们将原始密钥和加密的密钥一起保存。
要解密这个压缩文件,你需要先恢复密钥,然后使用相同的加密逻辑读取文件内容。
阅读全文
相关推荐
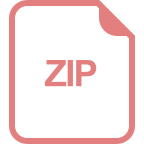
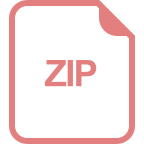

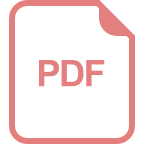
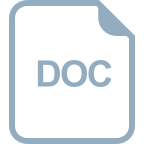
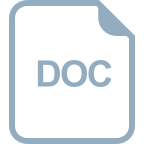
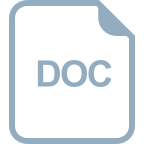





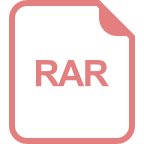
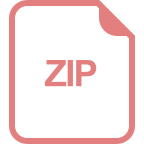
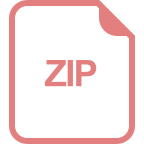
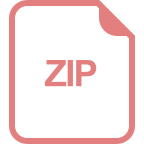
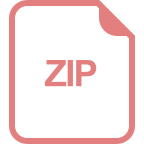
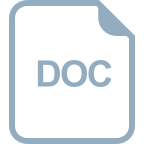