用C++完成以上程序
时间: 2024-09-18 07:00:35 浏览: 17
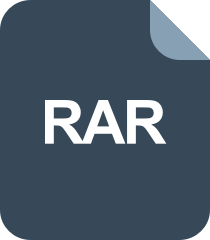
c++test_c++登录测试程序_
在C++中,你可以使用标准库中的`<string>`和`<algorithm>`来实现一个简单函数,用于计算字符串中的单词数量。下面是一个基本的例子:
```cpp
#include <iostream>
#include <string>
#include <cctype> // 包含转换字符类型的头文件
std::size_t countWords(const std::string& sentence) {
std::string cleanedSentence;
// 删除标点并转为小写
for (char c : sentence) {
if (ispunct(c)) { // 如果是标点符号
continue; // 跳过它
} else if (isalpha(c)) { // 如果是字母
cleanedSentence += tolower(c); // 添加到清理过的句子中并转为小写
}
}
// 使用find_if_not和count进行单词计数
return std::distance(sentence.begin(), std::find_if_not(cleanedSentence.begin(), cleanedSentence.end(), isspace));
}
int main() {
std::string input = "This is a sample sentence with some words.";
std::size_t wordCount = countWords(input);
std::cout << "The sentence has " << wordCount << " words.\n";
return 0;
}
```
在这个示例中,我们首先遍历输入字符串,删除所有的标点符号并将字母转换为小写。然后,我们使用`isspace`函数从清理后的句子中找到非空格的位置序列,最后返回这个序列的长度即为单词数量。
阅读全文
相关推荐
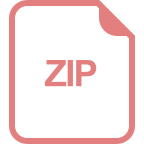
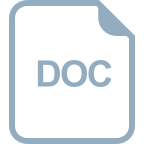
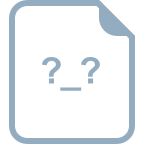
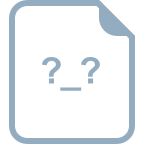
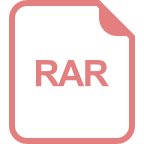
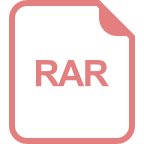
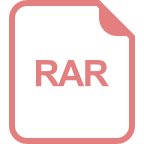
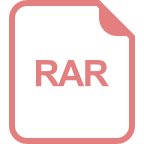
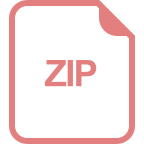
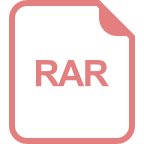
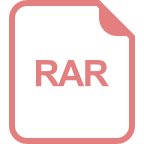
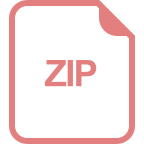
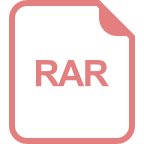
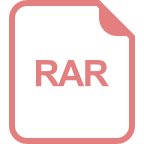