Python制作多线程下载器
时间: 2024-09-08 17:04:27 浏览: 121
在Python中制作多线程下载器通常会利用`threading`模块来实现并发下载,可以使用`requests`库来发送HTTP请求获取文件内容。下面是一个简单的例子,展示如何创建一个基本的多线程下载器:
```python
import requests
import threading
class DownloaderThread(threading.Thread):
def __init__(self, url, filename):
threading.Thread.__init__(self)
self.url = url
self.filename = filename
def run(self):
response = requests.get(self.url, stream=True)
with open(self.filename, 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
if chunk: # filter out keep-alive new chunks
file.write(chunk)
def download_parallel(urls, filenames, num_threads):
threads = []
for i in range(num_threads):
thread_url = urls[i]
thread_filename = filenames[i]
thread = DownloaderThread(thread_url, thread_filename)
threads.append(thread)
thread.start()
# 等待所有线程完成
for thread in threads:
thread.join()
# 使用示例
urls = ['http://example.com/file1', 'http://example.com/file2', 'http://example.com/file3']
filenames = ['file1.txt', 'file2.txt', 'file3.txt']
num_threads = min(len(urls), 5) # 设置最大线程数不超过URL数量的一半
download_parallel(urls, filenames, num_threads)
阅读全文
相关推荐
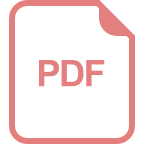
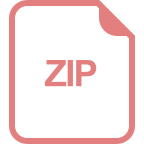
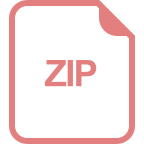
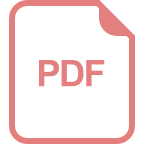
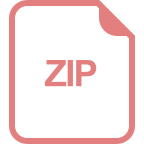
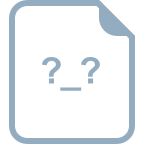
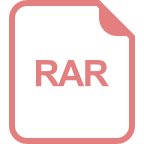
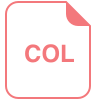
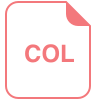





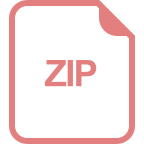