怎么判断变量的数据类型?
时间: 2023-09-28 10:10:02 浏览: 50
在 JavaScript 中,可以使用 `typeof` 运算符来判断变量的数据类型。`typeof` 运算符返回一个表示数据类型的字符串。
以下是一些常见的数据类型及其对应的 `typeof` 返回值:
- `undefined`:表示未定义的值。使用 `typeof` 判断时,返回 `'undefined'`。
- `boolean`:表示布尔值。使用 `typeof` 判断时,返回 `'boolean'`。
- `number`:表示数字。使用 `typeof` 判断时,返回 `'number'`。
- `string`:表示字符串。使用 `typeof` 判断时,返回 `'string'`。
- `symbol`:表示符号(ES6 新增)。使用 `typeof` 判断时,返回 `'symbol'`。
- `bigint`:表示大整数(ES2020 新增)。使用 `typeof` 判断时,返回 `'bigint'`。
- `object`:表示对象(包括数组、函数、日期等)。使用 `typeof` 判断时,返回 `'object'`。请注意,`typeof null` 返回 `'object'`,这是一个历史遗留问题。
- `function`:表示函数。使用 `typeof` 判断时,返回 `'function'`。
以下是使用 `typeof` 运算符判断变量数据类型的示例:
```javascript
const undefinedVariable = undefined;
console.log(typeof undefinedVariable); // 'undefined'
const booleanVariable = true;
console.log(typeof booleanVariable); // 'boolean'
const numberVariable = 42;
console.log(typeof numberVariable); // 'number'
const stringVariable = 'Hello';
console.log(typeof stringVariable); // 'string'
const symbolVariable = Symbol('foo');
console.log(typeof symbolVariable); // 'symbol'
const bigintVariable = BigInt(9007199254740991);
console.log(typeof bigintVariable); // 'bigint'
const objectVariable = { name: 'John' };
console.log(typeof objectVariable); // 'object'
const arrayVariable = [1, 2, 3];
console.log(typeof arrayVariable); // 'object'
const functionVariable = function() {};
console.log(typeof functionVariable); // 'function'
```
需要注意的是,`typeof` 运算符对于对象(包括数组和函数)返回的都是 `'object'`。如果需要更准确地判断对象的具体类型,可以使用其他方法,比如 `Array.isArray()` 判断是否为数组,`instanceof` 运算符判断是否为特定类型的实例等。
相关推荐
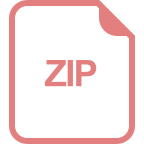
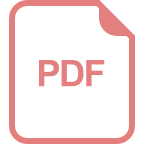
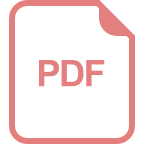














