java poi读取word
时间: 2023-09-04 20:14:59 浏览: 74
要使用Java POI库读取Word文档,您可以按照以下步骤进行操作:
1. 首先,您需要在项目中导入POI的依赖。如果使用Maven进行项目管理,可以在pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建一个`File`对象,指定要读取的Word文档的路径。
```java
File file = new File("path/to/your/word/document.docx");
```
3. 使用`FileInputStream`读取Word文档。
```java
FileInputStream fis = new FileInputStream(file);
```
4. 创建一个`XWPFDocument`对象,用于表示Word文档。
```java
XWPFDocument document = new XWPFDocument(fis);
```
5. 遍历文档中的段落和表格,并提取所需的内容。
```java
List<XWPFParagraph> paragraphs = document.getParagraphs();
for (XWPFParagraph paragraph : paragraphs) {
// 处理段落内容
String text = paragraph.getText();
// 进一步处理文本内容...
}
List<XWPFTable> tables = document.getTables();
for (XWPFTable table : tables) {
// 处理表格内容
List<XWPFTableRow> rows = table.getRows();
for (XWPFTableRow row : rows) {
// 处理行内容
List<XWPFTableCell> cells = row.getTableCells();
for (XWPFTableCell cell : cells) {
// 处理单元格内容
String text = cell.getText();
// 进一步处理文本内容...
}
}
}
```
6. 最后,记得关闭文件流。
```java
fis.close();
```
通过以上步骤,您可以使用Java POI库读取Word文档中的内容。请注意,POI库也可以读取旧版的`.doc`格式的Word文档,只需要将上述代码中的`XWPFDocument`替换为`HWPFDocument`即可。
相关推荐
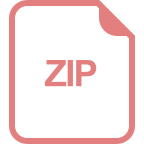
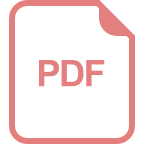
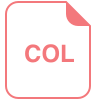
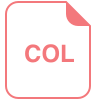
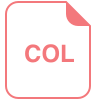
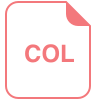
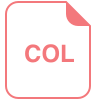









