MaxScript如何连接MySQL数据库?
时间: 2024-09-09 16:11:17 浏览: 83
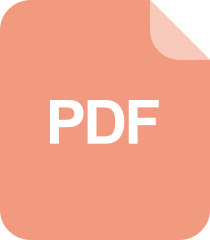
KEPSERVER如何连接MYSQL_.pdf
MaxScript是3ds Max的脚本语言,主要用于自动化3ds Max的操作和创建自定义用户界面。虽然MaxScript本身并不直接支持连接MySQL数据库,但可以通过调用外部脚本或程序来实现与MySQL数据库的连接。以下是一个简化的步骤说明:
1. 使用Windows的系统命令行执行MySQL客户端程序。可以通过MaxScript中的`dotnet`函数或者`system`函数执行一个外部命令,连接到MySQL数据库。
2. 你可以编写一个批处理文件(.bat)或者使用PowerShell脚本来执行MySQL命令行工具,例如:
```batch
@echo off
"C:\Program Files\MySQL\MySQL Server 8.0\bin\mysql" -u username -p password -D database_name -e "SQL语句"
```
或者在PowerShell中使用类似的命令。
3. 通过MaxScript调用这个批处理文件或PowerShell脚本,实现数据库操作。
示例MaxScript代码:
```maxscript
-- 调用系统命令行执行MySQL客户端命令
executeSystemCommand "path_to_your_batch_file.bat"
```
需要注意的是,这种方法取决于你的系统配置,包括MySQL客户端程序的安装路径,以及是否有必要的权限来执行这些操作。此外,如果3ds Max和MySQL不在同一台机器上,还需要通过网络协议来远程访问数据库。
阅读全文
相关推荐
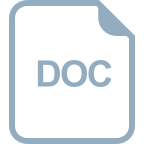
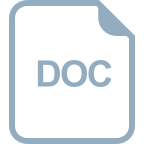
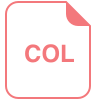
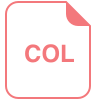
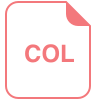
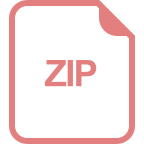
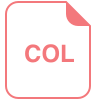
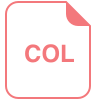
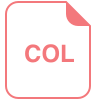
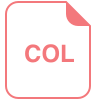
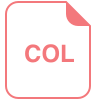
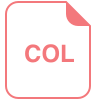
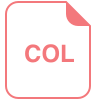
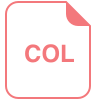
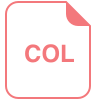
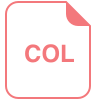

