编写一段程序,使用c语言和linux中文件夹相关系统调用对文件夹进行操作,实现文件夹复制的功能
时间: 2024-12-15 13:29:38 浏览: 19
在C语言中,要实现Linux下文件夹的复制功能,你需要使用POSIX标准库中的`unistd.h`头文件,特别是`mkdir()`, `chdir()`, `opendir()`, `readdir()`, 和`closedir()`函数来进行目录操作,以及`cp()`函数或者`rename()`函数进行文件复制。
以下是一个简单的示例,展示如何遍历源目录并逐个复制文件到目标目录:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
#define SRC_DIR "/path/to/source/directory"
#define DST_DIR "/path/to/destination/directory"
void copy_dir(const char* src_path, const char* dst_path) {
if (mkdir(dst_path, S_IRWXU | S_IRGRP | S_IXGRP | S_IROTH | S_IXOTH) != 0 && errno != EEXIST) {
perror("Failed to create destination directory");
return;
}
DIR* dir = opendir(src_path);
if (!dir) {
perror("Failed to open source directory");
return;
}
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) continue; // skip hidden files
char src_file[] = strncat(src_path, "/", sizeof(src_file) - 1);
char src_file_full[] = strncat(src_file, entry->d_name, sizeof(src_file_full) - 1);
char dst_file[] = strncat(dst_path, "/", sizeof(dst_file) - 1);
strcpy(dst_file, entry->d_name);
if (entry->d_type == DT_DIR) { // If it's a directory, recursively call copy_dir()
copy_dir(src_file_full, dst_file);
} else {
if (rename(src_file_full, dst_file) != 0) {
perror("Failed to rename file");
}
}
}
closedir(dir);
}
int main() {
if (copy_dir(SRC_DIR, DST_DIR) != 0) {
perror("Error copying directory");
return 1;
}
printf("Directory copied successfully.\n");
return 0;
}
```
在这个例子中,我们首先检查目标目录是否存在,如果不存在则创建。然后,打开源目录并逐个读取其内容。对于每个文件或子目录,我们会相应地处理它们的复制或递归复制。
注意,这只是一个基本示例,实际应用中你可能需要添加错误处理和权限检查等。
阅读全文
相关推荐
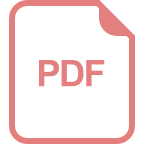
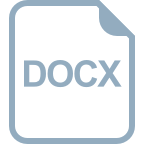
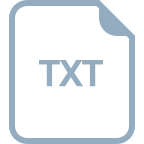















