C语言实现,遍历数组中每个元素,统计值相同的数据,返回其数量。
时间: 2024-10-15 09:13:37 浏览: 39
在C语言中,你可以通过创建一个函数来实现这个功能。首先,你需要定义一个结构体或者使用void指针来存储元素及其出现的次数。然后,我们可以编写一个循环来遍历数组,并在过程中更新计数。
这是一个简单的示例,假设我们有一个整型数组:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int value;
int count;
} ElementCount;
ElementCount* countOccurrences(int arr[], int size) {
// 初始化元素计数表
ElementCount *counts = malloc(size * sizeof(ElementCount));
for (int i = 0; i < size; i++) {
counts[i].value = arr[i];
counts[i].count = 1; // 初始计数为1
}
// 遍历数组并合并相同值的计数
for (int i = 0; i < size - 1; i++) {
for (int j = i + 1; j < size; j++) {
if (arr[i] == arr[j]) {
counts[j].count++; // 如果找到相同的值,增加计数
}
}
}
return counts;
}
// 打印结果
void printCounts(ElementCount *counts, int size) {
for (int i = 0; i < size; i++) {
printf("Value %d appears %d times.\n", counts[i].value, counts[i].count);
}
}
int main() {
int array[] = {1, 2, 3, 4, 5, 2, 3, 2, 1};
int size = sizeof(array) / sizeof(array[0]);
ElementCount *result = countOccurrences(array, size);
printCounts(result, size);
free(result); // 释放内存
return 0;
}
```
在这个示例中,`countOccurrences` 函数会统计数组中每个元素出现的次数,并返回一个指向`ElementCount`结构的动态数组。`printCounts`函数用于打印结果。记得在完成任务后释放动态分配的内存。
阅读全文
相关推荐









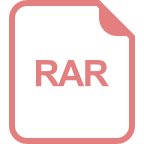

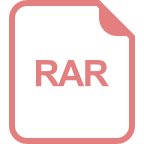





