python目标检测代码示例
时间: 2023-08-17 18:12:35 浏览: 104
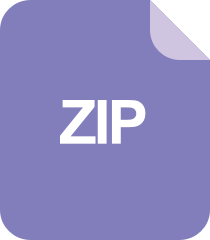
python目标检测

当然,下面是一个简单的 Python 目标检测代码示例,使用了 OpenCV 和 TensorFlow 的目标检测 API:
```python
import cv2
import numpy as np
import tensorflow as tf
# 加载模型
model_path = 'path_to_model/frozen_inference_graph.pb'
detection_graph = tf.Graph()
with detection_graph.as_default():
od_graph_def = tf.GraphDef()
with tf.gfile.GFile(model_path, 'rb') as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def, name='')
# 加载标签
label_map = 'path_to_labels/label_map.pbtxt'
categories = []
with open(label_map, 'r') as f:
lines = f.readlines()
for line in lines:
if 'name' in line:
category = line.split(':')[-1].strip().replace("'", "")
categories.append(category)
# 进行目标检测
def detect_objects(image):
with detection_graph.as_default():
with tf.Session(graph=detection_graph) as sess:
image_np_expanded = np.expand_dims(image, axis=0)
image_tensor = detection_graph.get_tensor_by_name('image_tensor:0')
boxes = detection_graph.get_tensor_by_name('detection_boxes:0')
scores = detection_graph.get_tensor_by_name('detection_scores:0')
classes = detection_graph.get_tensor_by_name('detection_classes:0')
num_detections = detection_graph.get_tensor_by_name('num_detections:0')
(boxes, scores, classes, num_detections) = sess.run(
[boxes, scores, classes, num_detections],
feed_dict={image_tensor: image_np_expanded})
# 显示检测结果
for i in range(int(num_detections[0])):
if scores[0][i] > 0.5:
class_index = int(classes[0][i])
class_name = categories[class_index - 1]
ymin, xmin, ymax, xmax = boxes[0][i]
xmin = int(xmin * image.shape[1])
xmax = int(xmax * image.shape[1])
ymin = int(ymin * image.shape[0])
ymax = int(ymax * image.shape[0])
cv2.rectangle(image, (xmin, ymin), (xmax, ymax), (0, 255, 0), 2)
cv2.putText(image, class_name, (xmin, ymin - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
return image
# 加载图像
image_path = 'path_to_image/image.jpg'
image = cv2.imread(image_path)
# 进行目标检测并显示结果
output_image = detect_objects(image)
cv2.imshow("Output", output_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个示例代码使用了一个已经训练好的目标检测模型(frozen_inference_graph.pb)和对应的标签文件(label_map.pbtxt)。你需要将模型路径和标签路径替换为你自己的路径,并提供一张待检测的图像(image.jpg)。
注意:这个示例代码仅仅是一个简单的演示,如果你想要在实际项目中进行目标检测,可能需要更复杂的模型和数据预处理。
阅读全文
相关推荐
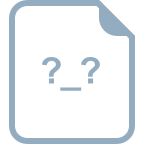
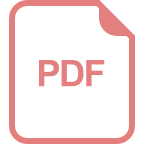
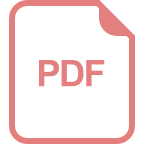
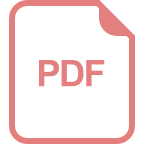
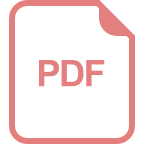
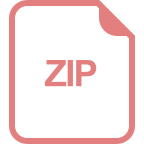
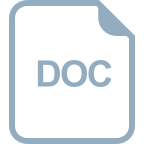
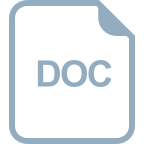

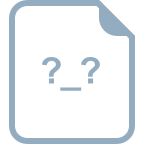
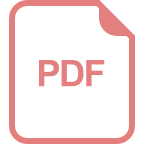
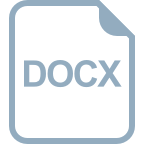
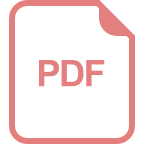
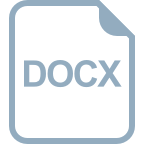
