python使用scp下载文件夹
时间: 2024-09-19 09:02:42 浏览: 55
在Python中,可以使用`paramiko`库来实现SCP(Secure Copy Protocol)协议,它是一个用于在网络上安全地传输文件的工具。如果你想要下载整个文件夹,首先需要安装`paramiko`库,可以使用pip安装:
```bash
pip install paramiko
```
然后你可以编写如下的Python代码来下载文件夹:
```python
import os
import paramiko
def download_folder_scp(source_path, target_path, username, host, port=22, password=None):
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
if password is not None:
client.connect(host, port=port, username=username, password=password)
else:
client.connect(host, port=port, username=username, pkey=None) # 使用SSH密钥对,如果没有密码
sftp = client.open_sftp()
remote_dir = '/' + source_path.lstrip('/') # 添加路径前缀,防止意外丢失路径信息
local_dir = target_path
try:
sftp.chdir(remote_dir)
os.makedirs(local_dir, exist_ok=True) # 创建本地目录如果不存在
for filename in sftp.listdir():
local_file = os.path.join(local_dir, filename)
sftp.get(filename, local_file)
print(f"文件夹 {remote_dir} 成功下载到本地 {local_dir}")
finally:
sftp.close()
client.close()
# 调用函数并提供远程主机、用户名、目标目录等信息
download_folder_scp('/path/to/source/folder', '/path/to/local/folder', 'your_username', 'your_host')
```
阅读全文
相关推荐
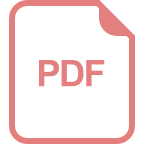
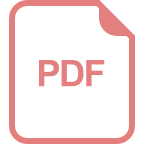
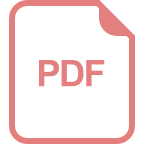

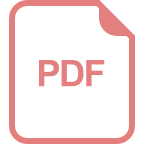
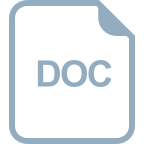
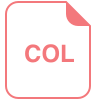
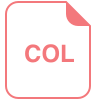
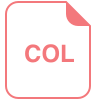






