python如何读取文件大小
时间: 2023-10-13 10:55:34 浏览: 165
您可以使用`os`模块中的`path`函数来获取文件的大小。下面是一个示例代码:
```python
import os
def get_file_size(filename):
size = os.path.getsize(filename)
return size
# 使用示例
file_path = 'path/to/your/file.txt'
file_size = get_file_size(file_path)
print(f"File size: {file_size} bytes")
```
在上面的代码中,`get_file_size`函数接受文件路径作为参数,并使用`os.path.getsize`函数获取文件的大小。返回的大小以字节为单位。您可以将文件路径替换为您要读取的实际文件路径。
相关问题
如何利用python读取文件大小
在Python中,你可以使用内置的`os`模块来读取文件的大小,即其字节数。以下是两种常见的方法:
1. **直接读取**:
```python
import os
def get_file_size(file_path):
try:
return os.path.getsize(file_path)
except FileNotFoundError:
print(f"{file_path} 文件不存在")
return None
file_size = get_file_size("your_file.txt") # 替换为你要读取的文件路径
```
`os.path.getsize()`函数会返回指定文件的字节大小,如果文件不存在则会抛出`FileNotFoundError`异常。
2. **使用with语句**:
```python
with open("your_file.txt", 'rb') as f: # 'rb'表示二进制读模式
file_size = f.seek(0, 2) # seek to end of the file
file_size = f.tell() # tell() returns current position which is now the file size
```
这种方法首先打开文件,然后将光标移动到文件末尾(`seek(0, 2)`),最后返回当前位置即文件大小。
记得替换`"your_file.txt"`为实际需要读取的文件路径。
python读取文件中的数字比较大小
Python读取文件中的数字比较大小可以通过以下步骤实现:
1. 打开文件并读取文件内容。
2. 将文件内容转换为数字列表。
3. 使用Python内置函数max()和min()比较数字列表中的最大值和最小值。
下面是一个示例代码,演示如何读取文件中的数字并比较大小:
```
with open('file.txt', 'r') as f:
content = f.read()
numbers = [int(num) for num in content.split()]
max_num = max(numbers)
min_num = min(numbers)
print("最大值为:", max_num)
print("最小值为:", min_num)
```
请注意,这个示例代码假设文件中只包含数字,每个数字之间用空格分隔。如果文件中包含其他内容,需要根据实际情况进行修改。
阅读全文
相关推荐
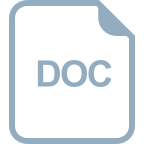
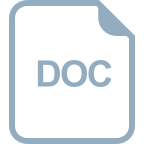
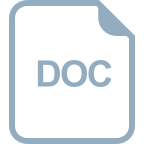
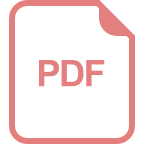
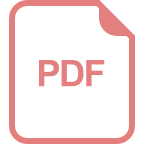
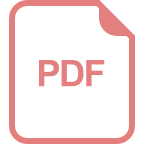
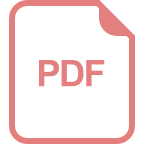
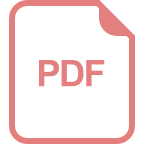
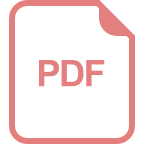
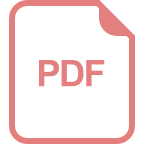
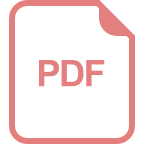
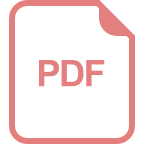




