winfrom 下载ftp文件
时间: 2023-07-13 09:03:08 浏览: 170
### 回答1:
在WinForm中下载FTP文件,首先需要使用System.Net命名空间中的WebClient类。以下是一个简单的步骤:
1. 在WinForm的代码文件中创建一个按钮,以便当用户点击该按钮时进行下载操作。
2. 在按钮的点击事件处理程序中,创建一个WebClient实例,并为其指定要下载的FTP文件的URL。
```csharp
private void DownloadButton_Click(object sender, EventArgs e)
{
WebClient webClient = new WebClient();
string ftpFileUrl = "ftp://example.com/ftpfile.txt";
}
```
3. 设置FTP服务器的登录凭据(如果需要进行身份验证)。
```csharp
webClient.Credentials = new NetworkCredential("username", "password");
```
4. 指定文件的本地保存路径。
```csharp
string localFilePath = "C:\\path\\to\\save\\file.txt";
```
5. 使用DownloadFileAsync方法开始异步下载操作。
```csharp
webClient.DownloadFileAsync(new Uri(ftpFileUrl), localFilePath);
```
6. 在需要的地方处理下载完成或下载错误的事件。
```csharp
webClient.DownloadFileCompleted += WebClient_DownloadFileCompleted;
webClient.DownloadProgressChanged += WebClient_DownloadProgressChanged;
```
```csharp
private void WebClient_DownloadFileCompleted(object sender, AsyncCompletedEventArgs e)
{
if (e.Error != null)
{
// 处理下载错误
}
else
{
// 下载完成后的操作
}
}
private void WebClient_DownloadProgressChanged(object sender, DownloadProgressChangedEventArgs e)
{
// 处理下载进度变化(可选)
}
```
通过这些步骤,你可以在WinForm中实现下载FTP文件的功能。
### 回答2:
在WinForm中下载FTP文件可通过使用System.Net命名空间下的FtpWebRequest类来实现。
首先,我们需要创建一个FtpWebRequest对象,并设置其属性来指定FTP服务器的地址、用户名和密码,以及请求的基本操作为下载。
```csharp
FtpWebRequest request = (FtpWebRequest)WebRequest.Create("ftp://服务器地址/文件路径");
request.Method = WebRequestMethods.Ftp.DownloadFile;
request.Credentials = new NetworkCredential("用户名", "密码");
```
然后,我们可以使用response对象来获取FTP服务器的响应,并从中获取下载的文件内容。
```csharp
FtpWebResponse response = (FtpWebResponse)request.GetResponse();
Stream responseStream = response.GetResponseStream();
StreamReader reader = new StreamReader(responseStream);
string fileContent = reader.ReadToEnd();
reader.Close();
response.Close();
```
最后,我们可以将文件内容保存到本地磁盘上指定的路径下。
```csharp
File.WriteAllText("本地路径", fileContent);
```
综上所述,这样就可以在WinForm中通过使用FtpWebRequest类来下载FTP文件了。请注意,由于网络请求可能会较慢,建议将文件下载的过程放在一个后台线程中执行,以避免阻塞界面的操作。
### 回答3:
在WinForms中下载FTP文件,可以通过使用C#编写代码来实现。首先,我们需要引用System.Net命名空间以便使用FTP相关的类和方法。
下面是一个简单的示例代码,说明了如何在WinForms中下载FTP文件:
```csharp
using System;
using System.IO;
using System.Net;
using System.Windows.Forms;
namespace FTP下载程序
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
// FTP服务器的地址和登录凭据
string ftpUrl = "ftp://example.com/file.txt";
string username = "username";
string password = "password";
// 本地保存下载文件的路径
string localFilePath = "C:\\file.txt";
// 创建FTP请求对象
FtpWebRequest request = (FtpWebRequest)WebRequest.Create(ftpUrl);
request.Method = WebRequestMethods.Ftp.DownloadFile;
request.Credentials = new NetworkCredential(username, password);
// 获取FTP响应对象
using (FtpWebResponse response = (FtpWebResponse)request.GetResponse())
{
// 打开本地文件流
using (Stream stream = response.GetResponseStream())
{
using (FileStream fileStream = new FileStream(localFilePath, FileMode.Create))
{
// 将FTP响应流写入本地文件流
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = stream.Read(buffer, 0, buffer.Length)) > 0)
{
fileStream.Write(buffer, 0, bytesRead);
}
}
}
}
MessageBox.Show("文件下载完成!");
}
}
}
```
在此示例中,我们首先在`Form1_Load`事件中指定了FTP服务器的地址`ftpUrl`,以及用于登录的用户名和密码。然后,我们创建了一个`FtpWebRequest`对象,设置请求方法为下载文件并提供登录凭据。
接下来,我们使用`request.GetResponse()`方法来获取FTP服务器的响应对象`response`。然后,我们使用`response.GetResponseStream()`方法获取FTP服务器的响应流,并打开本地文件流。最后,我们使用`stream.Read`和`fileStream.Write`方法将响应流中的数据写入本地文件。
在文件下载完成后,我们使用`MessageBox.Show`方法显示一个提示消息。
请注意,本示例代码仅适用于单个文件的下载。如果需要下载多个文件或实现其他FTP功能,可能需要进一步的代码调整。
阅读全文
相关推荐

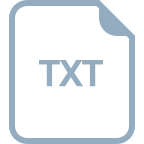
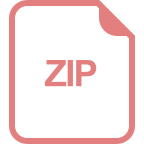
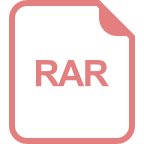
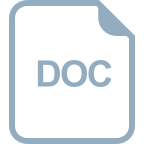
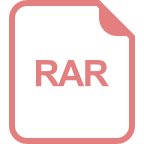
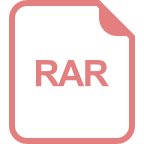
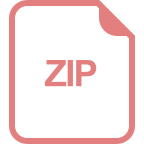
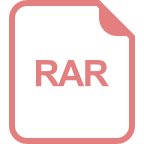
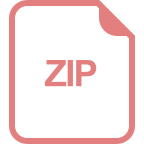
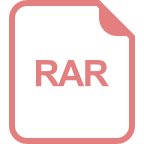
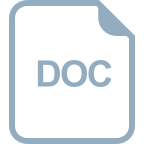
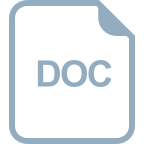
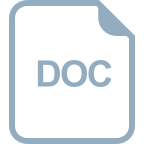
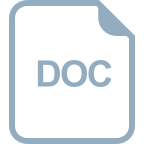
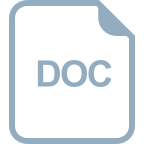