用C#语言实现模拟太阳系八大行星天体运动。
时间: 2024-12-06 21:23:42 浏览: 10
在C#中,实现模拟太阳系八大行星天体运动可以利用物理学的基本原理,特别是牛顿的万有引力定律。以下是简单的步骤:
1. **类设计**:
- 制定一个`行星`类作为`天体`的子类,添加特定于行星的数据,比如名称和公转周期。
2. **引力计算**:
- 实现一个`计算引力`方法,接收两个天体对象并基于它们的质量、距离和万有引力常数计算引力影响。
3. **运动更新**:
- 对于每个行星,定义一个`updateMotion()`方法,它会应用其他所有行星对其施加的引力,并根据收到的引力调整其速度和位置。这通常通过欧拉法或更精确的时间积分算法(如四阶龙格-库塔方法)来完成。
4. **主循环**:
- 使用`while`循环,定期(例如每秒或每一小时)更新所有行星的位置。可以使用`DateTime`和`TimeSpan`类来处理时间流逝。
5. **可视化**:
- 如果需要,你可以使用Windows Forms或Unity等图形库来展示行星的运动轨迹。
```csharp
// 示例代码
class Planet : CelestialBody
{
public double OrbitalPeriod { get; set; }
public void UpdateMotion(List<CelestialBody> otherBodies)
{
foreach (CelestialBody body in otherBodies)
{
if (body != this)
ApplyGravitationalForce(body);
}
// 根据时间间隔移动行星
//...
}
}
public class CelestialBody
{
public Vector3 Position { get; set; }
// 其他属性...
public void ApplyGravitationalForce(CelestialBody otherBody)
{
// 引力计算
}
}
public static void Main()
{
List<CelestialBody> planets = new List<Planet>();
planets.Add(new Planet { Name = "Mercury", ... });
planets.Add(new Planet { Name = "Venus", ... });
// 模拟运行并更新行星位置
while (true)
{
foreach (Planet planet in planets)
planet.UpdateMotion(planets);
// 更新UI显示
}
}
```
阅读全文
相关推荐






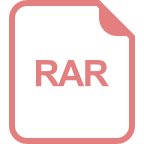











