h5+vue3 实现gps定位的详细代码
时间: 2024-12-12 16:28:31 浏览: 12
在H5和Vue3中实现GPS定位,通常会利用HTML5的Geolocation API以及Vue的响应式特性来获取用户位置。下面是一个简单的示例代码:
首先,安装依赖:
```bash
npm install axios vue-gmap geolocation
```
然后在`main.js`或你的组件中引入并配置Vue-GMap插件:
```javascript
import Vue from 'vue'
import { Gmap } from 'vue-gmap'
import Geolocation from 'vue-geolocation'
Vue.use(Gmap)
Vue.use(Geolocation)
// 使用Vue-Gmap的Google Maps API key
Vue.prototype.$gmapkey = 'YOUR_GOOGLE_MAPS_API_KEY'
```
接下来,在一个组件中使用地理定位功能:
```html
<template>
<div>
<g-map :center="mapCenter" :zoom="zoom">
<g-marker v-if="markerLocation" :position="markerLocation.position" @click="showInfoWindow"></g-marker>
<info-window ref="infoWindow" :open="infoWindowOpen" :options="{ pixelOffset: [0, -30] }">
<span>Your current location: {{ markerLocation ? markerLocation.address : "Loading..." }}</span>
</info-window>
</g-map>
<button @click="getLocation">Get Location</button>
</div>
</template>
<script>
export default {
data() {
return {
mapCenter: { lat: 37.7749, lng: -122.4194 }, // 初始化地图中心点(旧金山)
zoom: 8,
markerLocation: null,
infoWindowOpen: false,
};
},
methods: {
getLocation() {
this.geolocation.getCurrentPosition().then((pos) => {
const { latitude, longitude } = pos.coords;
this.mapCenter = { lat: latitude, lng: longitude };
this.markerLocation = { position: { lat: latitude, lng: longitude }, address: `Latitude: ${latitude}, Longitude: ${longitude}` };
}).catch((error) => {
console.error('Error getting location', error);
});
},
showInfoWindow() {
this.infoWindowOpen = !this.infoWindowOpen;
},
},
};
</script>
```
在这个例子中,当点击“Get Location”按钮时,会请求用户的当前位置,并更新地图中心、标记位置和信息窗口内容。
阅读全文
相关推荐
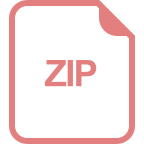
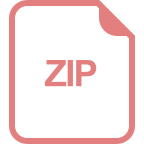
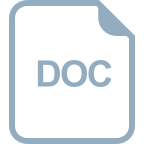
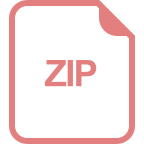
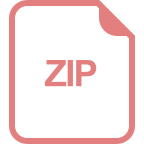
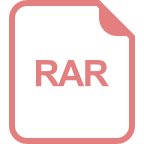
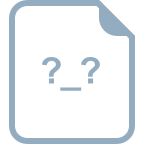
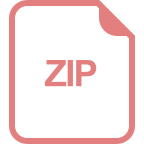
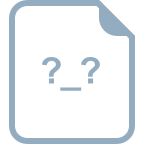
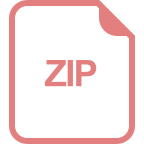
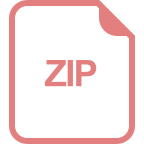
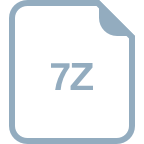
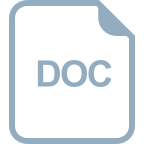
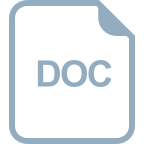
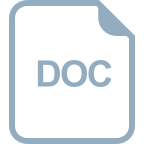
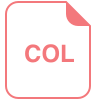
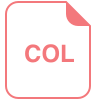