C#通过基本认证连接url官方示例
时间: 2024-10-16 20:10:57 浏览: 30
在C#中,你可以使用HttpClient类来通过HTTP基本认证(Basic Authentication)连接URL。下面是一个简单的示例:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class BasicAuthenticationExample
{
private const string USERNAME = "your_username";
private const string PASSWORD = "your_password";
public async Task ConnectWithBasicAuth(string url)
{
// 创建HttpClient实例
var httpClient = new HttpClient();
try
{
// 设置Authorization头,包含Base64编码的用户名和密码
byte[] credentialsBytes = System.Text.Encoding.UTF8.GetBytes($"{USERNAME}:{PASSWORD}");
string encodedCredentials = Convert.ToBase64String(credentialsBytes);
httpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", encodedCredentials);
// 发送GET请求并获取响应
HttpResponseMessage response = await httpClient.GetAsync(url);
if (response.IsSuccessStatusCode)
{
Console.WriteLine($"Connected to {url} with basic auth.");
Console.WriteLine(await response.Content.ReadAsStringAsync());
}
else
{
Console.WriteLine($"Failed to connect to {url}, status code: {response.StatusCode}");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
}
public static void Main()
{
string targetUrl = "http://example.com"; // 替换为你需要认证的URL
BasicAuthenticationExample example = new BasicAuthenticationExample();
example.ConnectWithBasicAuth(targetUrl).Wait(); // 异步阻塞等待任务完成
}
}
```
在这个例子中,你需要替换`USERNAME`和`PASSWORD`为实际的用户名和密码。记得确保你的代码在安全环境下运行,尤其是处理敏感信息时。
阅读全文
相关推荐



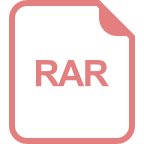

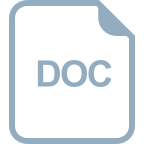












