立可得项目,获取商圈三个月内销售量前十的商品,用mybatis,在serviceimpl层怎么写
时间: 2024-04-16 14:24:11 浏览: 13
在使用 MyBatis 进行数据库操作的 ServiceImpl 层中,你可以按照以下步骤来实现获取商圈三个月内销售量前十的商品:
1. 首先,在 ServiceImpl 类中引入需要的包和注解:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.example.mapper.ProductMapper;
```
2. 在 ServiceImpl 类上使用 `@Service` 注解进行标记,使其成为 Spring 容器中的 Bean:
```java
@Service
public class YourServiceImpl implements YourService {
// ...
}
```
3. 在 ServiceImpl 类中注入 ProductMapper:
```java
@Autowired
private ProductMapper productMapper;
```
4. 编写一个方法来获取商圈三个月内销售量前十的商品:
```java
public List<Product> getTop10ProductsInBusinessCircle() {
// 获取当前时间
Date currentDate = new Date();
// 计算三个月前的日期
Calendar calendar = Calendar.getInstance();
calendar.setTime(currentDate);
calendar.add(Calendar.MONTH, -3);
Date threeMonthsAgo = calendar.getTime();
// 调用 ProductMapper 中定义的方法来查询销售量前十的商品
List<Product> top10Products = productMapper.getTop10ProductsInBusinessCircle(threeMonthsAgo, currentDate);
return top10Products;
}
```
5. 在 ProductMapper 接口中定义对应的查询方法:
```java
public interface ProductMapper {
List<Product> getTop10ProductsInBusinessCircle(Date startDate, Date endDate);
}
```
6. 在 MyBatis 的映射文件中编写对应的 SQL 查询语句,根据实际情况进行查询和排序:
```xml
<select id="getTop10ProductsInBusinessCircle" resultType="com.example.entity.Product">
SELECT *
FROM products
WHERE sale_date BETWEEN #{startDate} AND #{endDate}
ORDER BY sales_volume DESC
LIMIT 10
</select>
```
以上是一个简单的示例,根据你的实际情况进行调整。希望对你有所帮助!
相关推荐
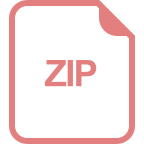
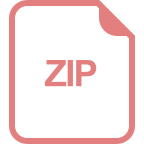
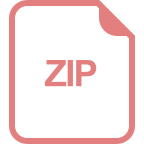
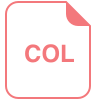










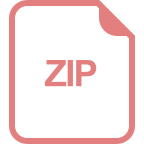
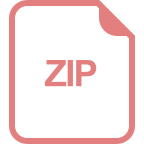
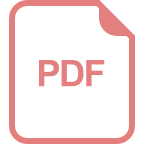
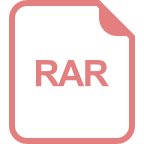