遗传算法和神经网络的代码
时间: 2024-08-15 16:01:58 浏览: 54
遗传算法是一种基于生物进化原理的优化搜索技术,主要用于解决复杂问题求解。其基本流程包括编码、选择、交叉和变异等步骤。在Python中,可以使用`deap`库来实现遗传算法,例如:
```python
from deap import base, creator, tools
# 创建种群
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
def eval(individual):
# 这里是一个评估函数,根据个体的表现计算适应度值
pass
toolbox = base.Toolbox()
toolbox.register("individual", tools.initRepeat, creator.Individual, choice)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("evaluate", eval)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutShuffleIndexes, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
pop = toolbox.population(n=100) # 初始化一个包含100个个体的种群
for gen in range(50): # 进行50代迭代
offspring = toolbox.select(pop, len(pop)) # 选择优秀的个体繁殖
offspring = [toolbox.clone(ind) for ind in offspring] # 复制避免直接修改原种群
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < 0.5:
toolbox.mate(child1, child2) # 交叉
del child1.fitness.values
del child2.fitness.values
for mutant in offspring:
if random.random() < 0.1:
toolbox.mutate(mutant) # 变异
del mutant.fitness.values
invalids = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = map(toolbox.evaluate, invalids)
for ind, fit in zip(invalids, fitnesses):
ind.fitness.values = fit
pop[:] = offspring # 更新种群
```
神经网络代码通常使用深度学习框架如TensorFlow或PyTorch编写。这里是一个简单的全连接神经网络例子(使用PyTorch):
```python
import torch.nn as nn
class NeuralNetwork(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(NeuralNetwork, self).__init__()
self.fc1 = nn.Linear(input_size, hidden_size)
self.relu = nn.ReLU()
self.fc2 = nn.Linear(hidden_size, output_size)
def forward(self, x):
out = self.fc1(x)
out = self.relu(out)
out = self.fc2(out)
return out
# 使用示例
model = NeuralNetwork(input_size=784, hidden_size=128, output_size=10)
input_data = torch.randn(1, 784)
output = model(input_data)
```
阅读全文
相关推荐






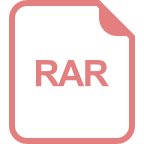




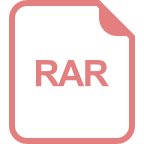




