qt读取数据并写入excel被覆盖
时间: 2024-10-20 14:12:09 浏览: 32
Qt是一个强大的跨平台应用程序开发框架,其中可以使用QSpreadsheetModel和QFileDialog等工具库来处理Excel文件(通常通过XLS或XLSX格式)。当你想要从Excel文件读取数据并将其覆盖到同一文件中,你可以按照以下步骤操作:
1. 导入所需的库:
```cpp
#include <QtWidgets>
#include <QtGui>
#include <QFile>
#include <QDesktopServices>
#include <QSpreadsheetModel>
```
2. 创建QSpreadsheetModel实例,并加载现有Excel文件:
```cpp
QFile file("path_to_your_excel_file.xlsx");
if (!file.open(QIODevice::ReadOnly | QIODevice::Text)) {
qDebug() << "Error opening the file";
return;
}
QSpreadsheetModel model(&file);
```
3. 获取工作表数据并存储到列表或其他容器中:
```cpp
const int rowCount = model.rowCount();
const int columnCount = model.columnCount();
QStringList data;
for (int row = 0; row < rowCount; ++row) {
for (int col = 0; col < columnCount; ++col) {
data.append(model.value(row, col).toString());
}
// Add a newline or separator between rows if needed
}
```
4. 清理旧数据,然后写入新数据(如果需要删除原有内容):
```cpp
model.removeRows(0, rowCount); // 删除所有行
model.setRowCount(0); // 或者仅设置行数为0,避免清空
// 现在将你的数据写回模型
for (int i = 0; i < data.count(); ++i) {
model.setData(model.index(i, 0), data.at(i)); // 写入第一列
}
```
5. 保存修改后的Excel文件:
```cpp
file.close();
QFile newFile("path_to_your_excel_file.xlsx");
if (!newFile.open(QIODevice::WriteOnly | QIODevice::Text)) {
qDebug() << "Failed to open the file for writing.";
return;
}
model.writeToStream(newFile);
newFile.close();
```
6. 异常处理和最终关闭:
```cpp
catch (const Exception &e) {
qDebug() << "An error occurred: " << e.what();
}
QMessageBox::information(nullptr, tr("Save Successful"), tr("Data has been written to Excel file."));
```
阅读全文
相关推荐
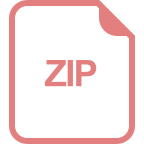
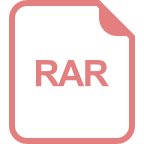
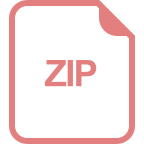
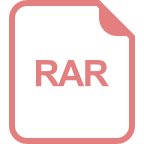



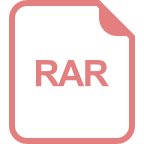
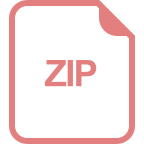
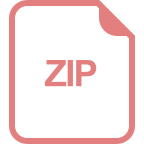
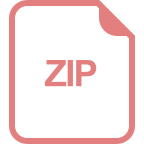
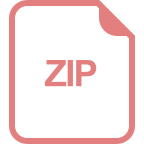





