Unity某个物体Destroy之后执行代码如何实现
时间: 2024-10-23 11:04:26 浏览: 22
在Unity中,当需要在一个游戏物体被销毁(Destroy()函数调用)后执行一些清理或逻辑处理的代码,你可以利用C#的委托(Delegate)和事件(Event)机制或者Coroutine来实现。这里有两个常用的方法:
1. 使用委托和事件:
- 首先,为那个即将被销毁的游戏对象添加一个自定义的“OnDestroy”事件。
```csharp
public class TargetObject : MonoBehaviour
{
public delegate void OnDestroyEventHandler();
public event OnDestroyEventHandler OnDestroy;
private void OnDestroy()
{
if (OnDestroy != null)
OnDestroy.Invoke();
}
```
- 然后,在需要执行后续操作的地方,订阅这个事件,并提供执行代码的方法。
```csharp
void Start()
{
TargetObject target = GetComponent<TargetObject>();
target.OnDestroy += OnDestroyLogic;
}
private void OnDestroyLogic()
{
// 清理或逻辑处理代码
}
```
2. 使用Coroutine:
- 当游戏对象即将销毁时,你可以存储一个引用并启动一个新的Coroutine等待销毁完成后再执行后续任务。
```csharp
private IEnumerator OnDestroyCoroutine()
{
yield return new WaitUntil(() => Destroy(gameObject)); // 等待游戏物体被销毁
// 清理或逻辑处理代码
}
private void OnDestroy()
{
StartCoroutine(OnDestroyCoroutine());
}
```
无论选择哪种方法,记得要在合适的时候取消订阅或停止Coroutine,避免内存泄漏。
阅读全文
相关推荐
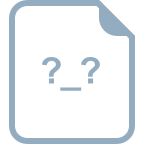
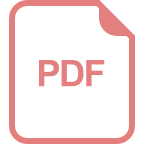
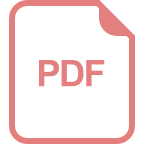

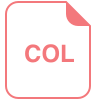
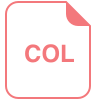
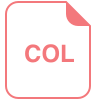
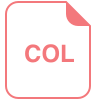

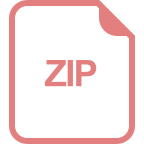
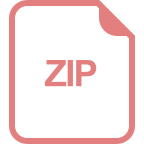
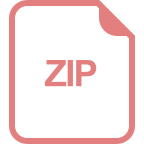
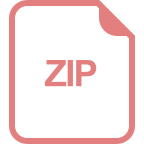
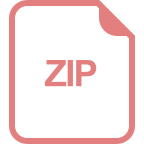
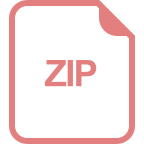
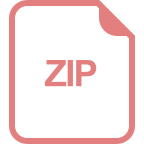
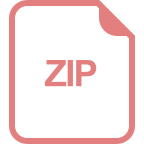