c#中ToUInt16和ToInt16区别
时间: 2023-09-28 09:06:11 浏览: 182
在C#中,`ToUInt16`和`ToInt16`是用于将数据转换为16位无符号整数(UInt16)和有符号整数(Int16)的方法。
`ToUInt16`方法用于将给定的数据转换为16位无符号整数(UInt16)类型。如果源数据小于0或大于UInt16的最大值(65535),则会抛出异常。示例代码如下:
```csharp
int intValue = 10;
ushort uintValue = Convert.ToUInt16(intValue);
```
`ToInt16`方法用于将给定的数据转换为16位有符号整数(Int16)类型。如果源数据超出Int16类型的范围(-32768到32767),则会抛出异常。示例代码如下:
```csharp
int intValue = 10;
short shortValue = Convert.ToInt16(intValue);
```
区别在于数据类型的符号和范围。`ToUInt16`将数据转换为无符号整数,范围为0到65535,而`ToInt16`将数据转换为有符号整数,范围为-32768到32767。
需要注意的是,如果要转换的数据超出目标类型的范围,无论是使用`ToUInt16`还是`ToInt16`,都会抛出异常。因此,在进行类型转换时,应该确保源数据在目标类型的有效范围内。
相关问题
C#中 10进制转16进制浮点数函数,IEEE-754标准浮点数
以下是C#中实现10进制转16进制浮点数函数的代码,其中使用了IEEE-754标准浮点数的转换方法:
```csharp
// 10进制转16进制浮点数函数
public static string DecimalToHexString(float num)
{
byte[] bytes = BitConverter.GetBytes(num);
string hexString = "";
for (int i = bytes.Length - 1; i >= 0; i--)
{
hexString += bytes[i].ToString("X2");
}
return hexString;
}
// IEEE-754标准浮点数转换方法
public static float ConvertIEEE754(string hexString)
{
uint num = Convert.ToUInt32(hexString, 16);
byte[] bytes = BitConverter.GetBytes(num);
float result = BitConverter.ToSingle(bytes, 0);
return result;
}
```
其中,DecimalToHexString函数将10进制浮点数转换为16进制字符串,而ConvertIEEE754函数将16进制字符串转换为IEEE-754标准浮点数。
如何在C#中解析接收到的PLC响应?
在C#中解析接收到的PLC响应通常依赖于PLC使用的通信协议。常见的工业协议如Modbus TCP、Modbus RTU或CANopen等都有特定的数据包结构。
以Modbus为例,假设你已经按照上述步骤获取了字节数组`receiveBuffer`,你可以这样做:
- **字节到整数的转换**:
```csharp
int slaveId = BitConverter.ToInt16(receiveBuffer, 0); // Modbus主设备地址通常在前两个字节
uint functionCode = BitConverter.ToUInt16(receiveBuffer, 2); // 功能码
```
- **读取寄存器值**(假如是请求读取输入寄存器):
```csharp
ushort startRegister = BitConverter.ToUInt16(receiveBuffer, 4);
uint length = BitConverter.ToUInt16(receiveBuffer, 6);
int[] data = new int[length / 2]; // 注意这里是按每个16位处理
for (int i = 0; i < length / 2; i++) {
data[i] = BitConverter.ToInt16(receiveBuffer, 8 + i * 2);
}
```
- **构建响应数据**(根据协议规则):
```csharp
byte[] response = new byte[8 + length];
Buffer.BlockCopy(BitConverter.GetBytes(slaveId), 0, response, 0, 2);
Buffer.BlockCopy(BitConverter.GetBytes(functionCode), 0, response, 2, 2);
Buffer.BlockCopy(BitConverter.GetBytes(startRegister), 0, response, 4, 2);
Buffer.BlockCopy(BitConverter.GetBytes(length), 0, response, 6, 2);
// 将数据复制到响应数据块的适当位置
Array.Copy(data, 0, response, 8, length);
```
这只是一个非常基础的例子,实际解析可能会更复杂,取决于具体的协议细节和PLC的具体配置。如果你不清楚PLC使用的协议,可能需要查阅相关的文档或使用专门的库来帮助解析。
阅读全文
相关推荐
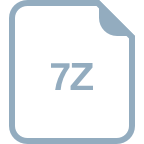
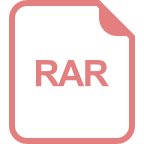
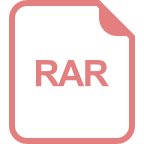
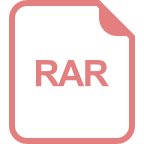
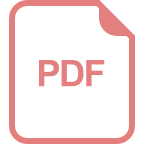
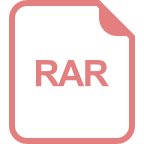
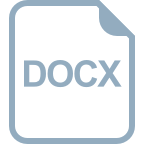
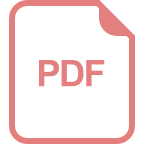
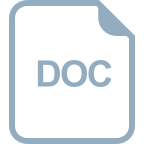
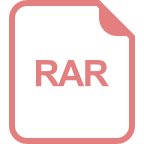
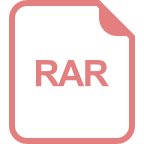
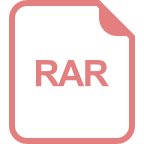
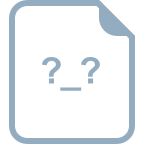
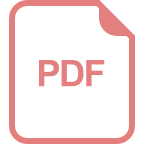
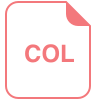
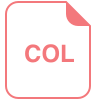
