维吉尼亚密码解密算法c语言
时间: 2024-10-05 11:02:55 浏览: 3
维吉尼亚密码(Vigenère Cipher),也称为加法表密码,是一种古典加密技术,利用凯撒密码的思想结合了简单的替换和移位。在C语言中实现维吉尼亚密码解密,你需要准备一个字母表,并通过一个密钥序列对每个明文字符进行多次循环移位。
以下是基本的步骤:
1. 定义一个字母表,通常包含所有大写字母。
2. 获取用户输入的密文、密钥以及字母表长度。
3. 创建一个空字符串用于存放解密后的文本。
4. 对于密文中的每一个字符,计算其基于字母表位置的索引加上密钥当前位置的值(取模字母表长度,防止溢出)。然后找到对应的新位置对应的字母作为解密结果。
5. 将解密后的字符添加到结果字符串中。
6. 移动密钥到下一个字符,重复步骤直到遍历完整个密文。
下面是一个简化的C语言代码示例:
```c
#include <stdio.h>
#include <string.h>
char alphabet[26] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
int keylen;
void decrypt(char* ciphertext, char* key) {
int i, j;
for (i = 0; ciphertext[i]; i++) {
int c_index = ciphertext[i] - 'A';
int k_index = key[i % keylen] - 'A';
// 解密公式: (ciphertext + k) % 26
int decrypted_index = (c_index + k_index) % 26;
ciphertext[i] = alphabet[decrypted_index] + 'A';
}
}
int main() {
char plaintext[100], ciphertext[100], key[100];
printf("Enter the ciphertext: ");
fgets(ciphertext, sizeof(ciphertext), stdin);
keylen = strlen(key);
printf("Enter the key: ");
fgets(key, sizeof(key), stdin);
// 去除换行符
ciphertext[strlen(ciphertext) - 1] = '\0';
key[strlen(key) - 1] = '\0';
decrypt(ciphertext, key);
printf("Decrypted text: %s\n", ciphertext);
return 0;
}
```
相关推荐
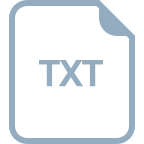
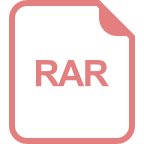
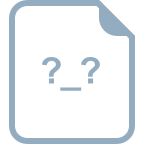














